When creating an Android app, every developer faces a lot of issues. And when thinking through the client-side, like an eye-catching design and usability, it’s also crucial to figure out what will be under the hood. The first and foremost question is how to store information. It is vital for every Android developer to choose the right database that will fit the product and not destroy it.
Guest blog post by Vitaly Kuprenko.
Vitaly is a technical writer at Cleveroad, a web and app development company in Ukraine. He enjoys telling about tech innovations and digital ways to boost businesses.
Popular solutions
When developers start looking for the best database, they can find a plethora of solutions, especially when they want to store all needed information on the server. But when data needs to be saved on user’s device, there are 2 major options that will come in handy. There are Realm and SQLite. Let's take a closer look at both databases.
SQLite
This embedded database remains the most widely utilized SQL DB engine. It was developed back in 2000 by Richard Hipp who also created Fossil version control system. SQLite is primarily geared toward storing all data on devices and moving away from common client-server architecture. Thousands of mobile apps were built using this tool.
Realm
This tool positions itself as a DB management system that can easily replace SQLite. It is absolutely free of charge. Realm appeared much later than SQLite. Its development began in 2010, its first name was TightDB. In 2014 its creators renamed it to Realm and released the beta version of their brainchild. Created from scratch, this tool works on its own persistence engine. Realm is widely used for mobile development, especially among newbies in Android development. This DB works seamlessly with Java, Swift, Objective-C, Xamarin, React Native.
Now, this tool continues to gain popularity among mobile developers. Let’s find out the main reasons why Realm now bypasses time-tested SQLite.
SQLite drawbacks
Even the most powerful tools have drawbacks. In case your project is not so complicated, you can opt for SQLite. This database perfectly fits small apps. Otherwise, you may face the following challenges:
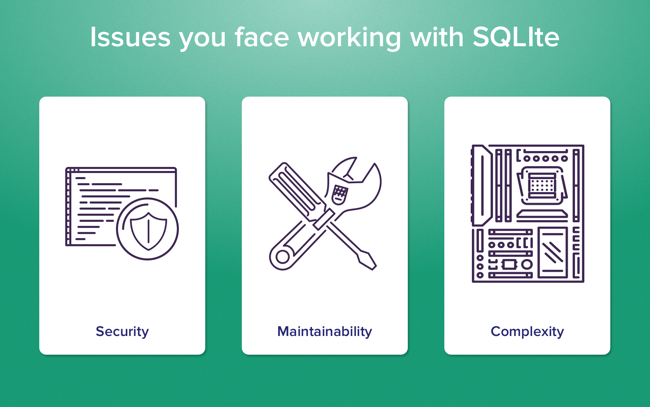
Scalability
There is no denying that the longer your app is on market, the more data need to be stored. If you want your application to work seamlessly with a big scope of data, it is a necessity to write complex queries. Newbie developers have problems with this because of a lack of experience with using SQL.
Another weak side is data migration. The first and foremost con is the inability to rename or delete the column in a few lines of code. When you eager to do the migration, you have to take several steps.
First, create a new table based on the old one. Then copy info from one table to another. After get rid of the old table and change the name of the new one. The process is quite similar if you want to add or delete constraints.
Security
Even though its long development process, SQLite still has a couple of security issues. Since security is of a great importance for any mobile application, you should definitely know about this issues before using SQLite.
1. Sometimes journal files are not deleted
Temporary journal files allow you to rollback when something went wrong. As a rule, they are kept for a certain period of time and deleted after a transaction. But sometimes they stay, and it may cause data corruption.
You may think that turning journal off can be a good solution. But bear in mind that in this case app crash will cause irreparable damage to the database.
2. SQLite DB is stored in a single file
Developers can put SQLite DB file wherever they want in the directory hierarchy. It may appear to be beneficial. But in practice, it is a high risk to lose data when any rogue process will open and rewrite the database file. To prevent that, protect the database on the file level. Don’t put this file into the web root and think twice when you set a file permission. To solve this problem, add several SQLite extensions or encrypt information right before it is put it on the journal.
Complexity
Experienced SQL coders will have no difficulties with solving upcoming problems. But when you start using SQLite for developing Android apps, applying this tool may turn into a nightmare. Massive structure and complexity of SQLite forced developers to search for a simpler alternative that won’t rest upon SQL. And here’s Realm comes to rescue.
Realm vs. SQLite
Appeared not long ago, Realm is a good alternative for SQLite. This tool is used by such big companies like IBM, CISCO, Amazon, Google, and Intel. Developers have already found several advantages of Realm over SQLite. Cleveroad has also tried out this brand new tool. And here are the results of our comparison:
1. What is easier to use?
In contrast to its predecessor, Realm is much easier. Consider the following examples. Assume you’ve chosen SQLite for your project. To create a table, add the following lines to your code:
private static final String SQL_CREATE_FAVOURITE = "CREATE TABLE " + FavouritesDBContract.FavouritesEntry.TABLE_NAME + " (" + FavouritesDBContract.FavouritesEntry._ID + INTEGER_TYPE + " PRIMARY KEY AUTOINCREMENT," +FavouritesDBContract.FavouritesEntry.COLUMN_NAME_TITLE + TEXT_TYPE + COMMA_SEP + FavouritesDBContract.FavouritesEntry.COLUMN_NAME_IMAGE_URL + TEXT_TYPE + " )";
public void onCreate(SQLiteDatabase db) {
db.execSQL(SQL_CREATE_FAVOURITE);
}
In case you opted for Realm, you should just inherit RealmObject.
public class Favourites extends RealmObject{
String title;
String imageLink;
@Nullable
ImageResponse image;
public Favourites(String title, String imageLink, @NullableImageResponse image) {
this.title = title;
this.imageLink = imageLink;
this.image = image;
}
}
Move forward to another task: create the query to see the list of results. Here is how the code looks like on SQLite.
@Override
public void getFavorites(int page, GetFavouritesCallBack callback) {
List<Favourites> favourites = new ArrayList<>();
try {
Cursor cursor = mDb.query(
FavouritesDBContract.FavouritesEntry.TABLE_NAME, null, null, null, null, null, null);
if (cursor != null && cursor.getCount() > 0) {
while (cursor.moveToNext()) {
String title = cursor.getString(cursor.getColumnIndexOrThrow(FavouritesDBContract.FavouritesEntry.COLUMN_NAME_TITLE));
String imagePath =cursor.getString(cursor.getColumnIndexOrThrow(FavouritesDBContract.FavouritesEntry.COLUMN_NAME_IMAGE_URL));
Favourites favourite = new Favourites(title, imagePath, null);
favourites.add(favourite);
}
}
} catch (IllegalStateException e) {
callback.onDataNotAvailable();
}
}
Code written on Realm is more laconic:
public List<Favourites> getFavorites() {
Realm realm = getRealm();
List<Favourites> favourites = realm.where(Favourites.class).findAll();
favourites = realm.copyFromRealm(favourites);
realm.close();
return favourites;
}
Return back to data migration. Previously we’ve mentioned how to perform it using SQLite. Now let’s find out how to do it with Realm. Use this code:
public class FavouritesMigration implements RealmMigration {
@Override
public void migrate(DynamicRealm realm, long oldVersion, long newVersion) {
RealmSchema schema = realm.getSchema();
if (oldVersion == 1) {
schema.get("Favourites").removeField("image");
oldVersion++;
}
}
}
And don’t forget to add a few lines in config:
private static final RealmConfiguration mConfig = new RealmConfiguration.Builder()
.schemaVersion(FAVOURITES_SCHEMA_VERSION)
.migration(new FavouritesMigration())
.build();
Apart from this, it is worth saying about the simplicity of the Realm’s implementation. It is ORM platform with a wide range of features. It is quite easy to save, read and add objects to the DB. A newbie Android developer will quickly puzzle out how to use this tool. What’s more, you need not think about internal collections. Realm creators have done it for you.
2. Which tool is faster?
Realm is also better than SQLite when we talk about speed. And this fact was proved by the research published on the Realm’s official website. It was stated that Realm is 10 times faster than the raw SQLite. For example, Realm allows inserting 20 thousand records in one second. SQLite allows inserting only 9 thousand records.
3. Which tool has better documentation?
Both tools have exceptionally good documentation. When you have questions about using one of these tools, you can go to official websites and see the documentation available online for everybody.
4. What about accessibility?
As for Realm, this tool allows developers to copy objects and still have access to them outside the Realm database. But this database has certain limitations. Its files cannot be accessed by several processes in parallel.
5. What extra features do they have?
Now Realm has a great variety of features that can facilitate the development process. For instance, this tool supports JSON file format. Data change notifications allow you to track every change in your database, while encryption support keeps user's data secure.
6. What about data synchronization?
Realm doesn't copy information. Instead, this database works on live objects. Queries allow getting a list of object references. Thus, it works on original Realm data. SQLite copies data from DB when a developer sends a query. So to save all changes, a developer should write them back to DB again.
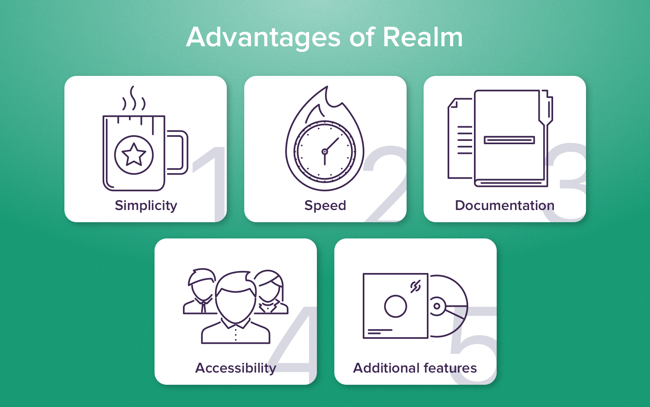
It is clear for now why more developers choose Realm for Android development. This simple and fast development tool can be used by both newbies and professionals in mobile development.
2017 witnessed the arrival of Room persistent library that became a real game changer on the competition between Realm and SQLite.
What is Room?
This library was introduced at Google I/O 2017. It is an abstraction layer over SQLite to solve several problems with this database. Room allows SQLite to catch up and overtake Realm in some cases.
- Room requires storage space of 50 KB while Realm’s size can reach 4MB. So when talking about size, Room is far ahead.
- Room enables using LiveData class without delays and interruptions.
- Problems with SQLite security we’ve mentioned above was partly solved by Room. Room enables developers to implement an SQLite extension which supports encryption. For instance, you can plug in SQLCipher.
- Room has finally solved the problem with data migration.
- Multithreading is an issue of Realm, while it is a commonplace for Room.
- Talking about method count, Realm uses approximately 2000 methods, while Room has only 300 in it. One point to Room!

Summing up
Each option listed above has both its strong and weak sides. SQLite can scare newbie developer by its complexity, that’s why more beginners use Realm for their first Android projects.
Realm can work smoothly without any additional settings, while SQLite may require a long time to choose the right configuration for the particular project. But when your projects will become more complex an large-scale, you will understand that using SQLite coupled with Room is a better option for you.
So when you choose between these two databases, primarily take into consideration the architecture of the project and the level of your coding skills.