Best Practices for Using Redis: Protect Your App, Reduce Costs, and Optimize Performance 2025
Redis is a powerful in-memory data store that enhances application performance, but improper usage can lead to security risks, high costs, and inefficiencies. In this guide, we’ll explore redis best practices that will help you protect your app, reduce costs, and maximize Redis efficiency.
1. Secure Your Redis Deployment
🔒 Enable Authentication and Authorization
By default, Redis has no authentication, making it vulnerable to unauthorized access. Secure it by enabling authentication:
requirepass "thisEveYs3cur3P@zzwork"
For cloud-based Redis (AWS ElastiCache, Redis Cloud), configure IAM roles and VPC restrictions.
🔒 Disable Remote Access
Restrict Redis to local access if not needed externally:
bind 127.0.0.1
If you must expose Redis, use TLS encryption and firewall rules.
🔒 Use Protected Mode
Ensure Redis is not exposed to the internet:
protected-mode yes
2. Optimize Memory Usage & Reduce Costs
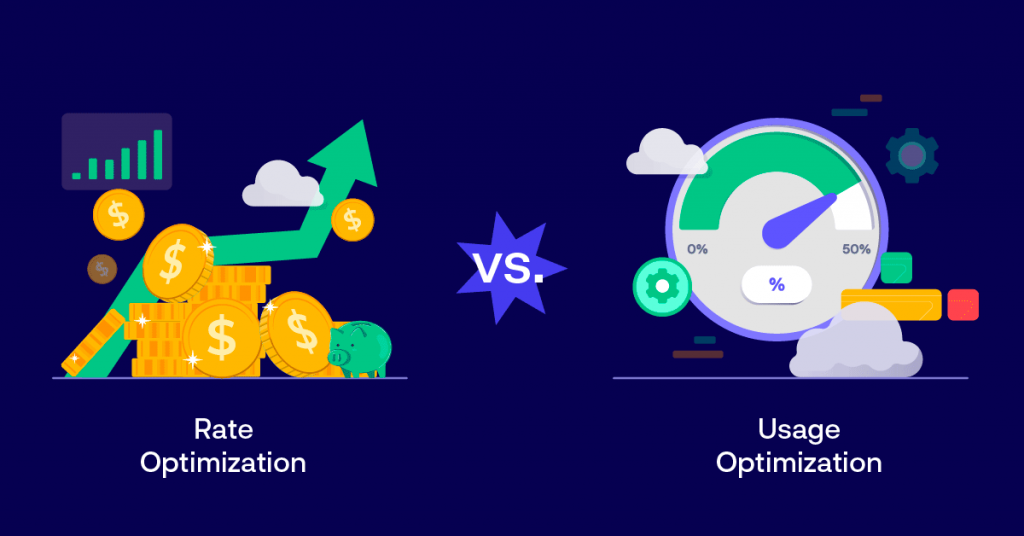
⚡ Use the Right Eviction Policy
When Redis is full, it must remove keys. Choose an efficient eviction policy:
maxmemory-policy allkeys-lru
allkeys-lru
(Best for caching) → Removes the least recently used keys.volatile-lru
(Only with TTL) → Removes LRU keys that have an expiration.noeviction
(Risky) → Prevents new writes when memory is full.
⚡ Set Expiration for Keys (TTL)
Prevent unnecessary memory usage by setting automatic expiration:
SET session:12345 "user_data" EX 3600 # Expires in 1 hour
For frequently changing data, use volatile-lru
to remove old keys dynamically.
⚡ Use Efficient Data Structures
Avoid storing inefficient data formats. Use:
- Hashes instead of multiple keys (
HSET user:123 name "John" age 30
) - Sets instead of lists for unique values
- Bitmaps & HyperLogLog for space-efficient counting
3. Improve Redis Performance
🚀 Use Connection Pooling
Reduce latency by reusing Redis connections instead of creating new ones:
const client = new Redis({
maxRetriesPerRequest: null,
enableReadyCheck: false,
});
🚀 Use Pipelining for Bulk Operations
Avoid multiple round-trips by sending batch commands:
const pipeline = client.pipeline();
pipeline.set("key1", "value1");
pipeline.set("key2", "value2");
pipeline.exec();
🚀 Avoid Large Keys & Values
- Keep keys short but meaningful (e.g.,
user:123:name
instead ofvery_very_long_user_id_name
) - Store compressed data when possible
- Avoid large JSON objects; instead, use hashes
🚀 Avoid Using KEYS Command in Production
Using KEYS *
or KEYS pattern
in production can cause performance degradation as it scans the entire dataset. Instead, use:
SCAN 0 MATCH pattern COUNT 100
This approach is non-blocking and avoids locking Redis during execution.
4. Ensure Data Persistence & Reliability
🔄 Choose the Right Persistence Mode
Redis offers two persistence methods:
- RDB (Snapshot-based, faster recovery):
save 900 1 # Save every 15 min if at least 1 key changed save 300 10 # Save every 5 min if at least 10 keys changed
- AOF (Append-only file, better durability):
appendonly yes appendfsync everysec # Syncs changes every second (balance between durability and performance)
always
→ Ensures maximum durability but can slow performance.everysec
(Recommended) → Syncs once per second for a good balance.no
→ Relies on OS to sync (least durable).
aof-use-rdb-preamble yes
optimizes recovery time by combining AOF and RDB.
For mission-critical apps, use both AOF + RDB.
🔄 Set Up Replication for High Availability
Enable master-replica for failover protection:
replicaof master_ip master_port
For cloud Redis, enable Cluster Mode or Read Replicas.
5. Monitor & Debug Redis Usage
📊 Monitor Memory & Performance
Use the following commands to analyze Redis usage:
redis-cli INFO memory # Check memory stats
redis-cli MONITOR # Debug real-time queries
Enable Redis Slow Log to find bottlenecks:
CONFIG SET slowlog-log-slower-than 10000 # Log queries taking over 10ms
SLOWLOG GET 10 # View last 10 slow queries
📊 Use Redis Keyspace Notifications
Get real-time alerts for Redis key changes:
CONFIG SET notify-keyspace-events KEA
Conclusion
Following these best practices will secure your Redis instance, optimize memory usage, improve performance, and ensure reliability. Whether you’re using Redis as a cache, message broker, or real-time database, these strategies will help you build a cost-effective and high-performance Redis setup.
📌 Need Help? Let me know in the comments how you manage Redis in production! 🚀
Post Comment
You must be logged in to post a comment.