Turning degrees into radians helps make math and programming calculations more accurate. In Python, knowing radians is important for trigonometry, making calculations smoother and more precise. Therefore, in this article, we are going to learn how to convert data from degrees to radians, discussing various methods with code. So, let’s get started!
What Are Radians and Degrees?
Degrees are the units we use to measure angles, much like how we tell time on a clock. A full circle is split into 360 parts, each called a degree. For example, if you turn a quarter of the way around a circle, you’ve turned 90 degrees.
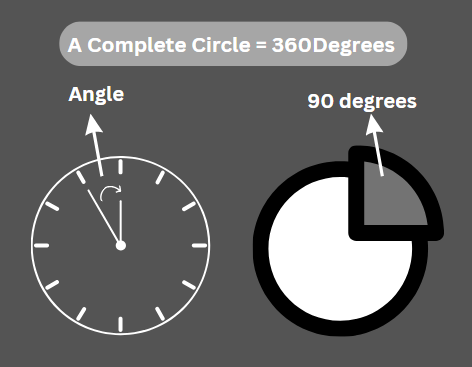
Radians are another way to measure angles, but they’re a bit different. Instead of dividing the circle into 360 parts like degrees, we measure angles in terms of the length of the arc of the circle that the angle cuts off. For example, if you walk along the edge of a circle with a radius of 1 meter, and you end up 1 meter away from where you started, you’ve walked an angle of 1 radian.
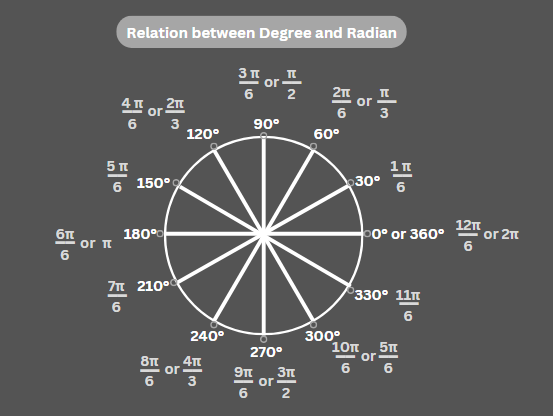
Here’s the key relationship between degrees and radians: There are 360 degrees in a full circle, and there are 2π radians in a full circle. So, 360 degrees = 2π radians.
We convert degrees to radians because radians are commonly used in math and science calculations. Trig functions like sine, cosine, and tangent work best with radians. Using radians makes calculations involving these functions simpler and more accurate. Radians are closely tied to basic math concepts like circles and angles. They show up in many physics and engineering equations because they fit naturally with these ideas.
Ways to Convert Degrees to Radians in Python
Now that we have seen the concepts of degrees and radians, let’s see how we can convert degrees into radians using Python. Carefully observe the following methods.
Method 1: Manual Calculation
We can use data and pass it into the formula to calculate the angle.
import math
d = float(input("Enter the angle in degrees: "))
r = d * (math.pi / 180)
print("Degrees:", d)
print("Converted into Radians:", r)
This code gets an angle in degrees from the user, converts it to radians using the formula, and prints both the original degrees and its equivalent in radians.
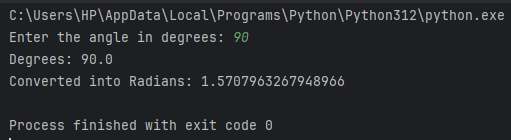
Method 2: By Using radians() Function
We can also achieve the results using the radians() function which is a part of the math library. Let’s see how.
import math
d = float(input("Enter the angle in degrees: "))
r = math.radians(d)
print("Degrees:", d)
print("Converted into Radians:", r)
This code gets an angle in degrees from the user, converts it to radians using the radians() function, and then prints both the original degrees and its equivalent in radians.
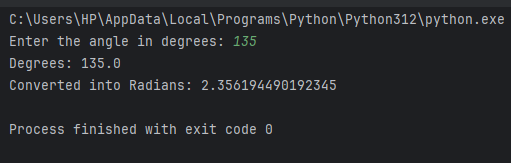
Method 3: By Using deg2rad() Function
In Python’s NumPy library, we have the deg2rad() function, which helps convert degrees to radians. It is one of the best ways to do so.
import numpy as np
d = float(input("Enter the angle in degrees: "))
r = np.deg2rad(d)
print("Degrees:", d)
print("Converted into Radians:", r)
This code takes an angle in degrees as input, converts it into radians using NumPy, and then prints both the original degrees and the converted radians.
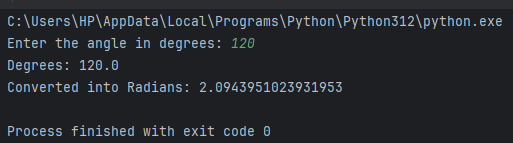
We can also aim for multiple angles by accepting array input for multiple data points. Like here:
import numpy as np
a_input = input("Enter the angles in degrees : ")
d = np.array([float(angle) for angle in a_input.split()])
r = np.deg2rad(d)
print("Degrees:", d)
print("Converted into Radians:", r)
The code splits the input into separate angles, converts each angle to a number, and stores them in an array. After that, it converts the angles from degrees to radians and prints both the original degrees and their corresponding radians.
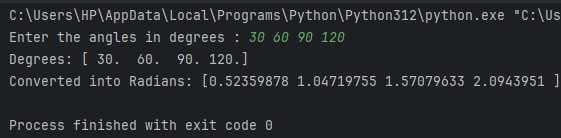
We can also convert radian angles into degrees using the rad2deg() function in Python. Let’s see how:
import numpy as np
a_input = input("Enter the angles in radians : ")
r = np.array([float(angle) for angle in a_input.split()])
d = np.rad2deg(r)
print("Radians:", r)
print("Converted into Degrees:", d)
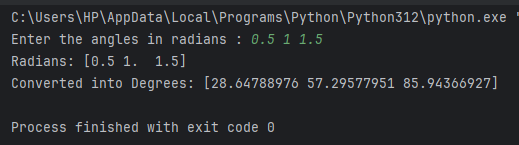
Conclusion
We have reached the end of this article. I hope the concepts of radians and degrees have fit well in your mind. In this article, we discussed three major ways of converting values given in degrees to radian angles. Additionally, we explored how to work with multiple values as well.
Do you know how to calculate cumulative sum or perform exponential operations in Python? Follow the links to learn more about them.
Reference
https://numpy.org/doc/stable/reference/generated/numpy.deg2rad.html