Rounding numbers means making them simpler by removing extra decimal parts. It’s important because it makes math easier and helps us understand and work with numbers better. In this article, let’s discuss how we can round off numbers using simple functions in Python’s NumPy. So let’s begin.
Rounding Numbers Using NumPy
1. The round() Function
The round() changes a number or a group of numbers to the nearest whole number or a certain number of decimal places.
The basic syntax looks like this:
np.round(array, decimals)
Here,
- array: This is the input array or number that you want to round.
- decimals: This allows you to choose how many numbers after the decimal point you want to round to. If you don’t say, it will round to the nearest whole number.
How it rounds:
If the decimal part of a number is less than 0.5, it rounds down. If the decimal part is 0.5 or greater, it rounds up.
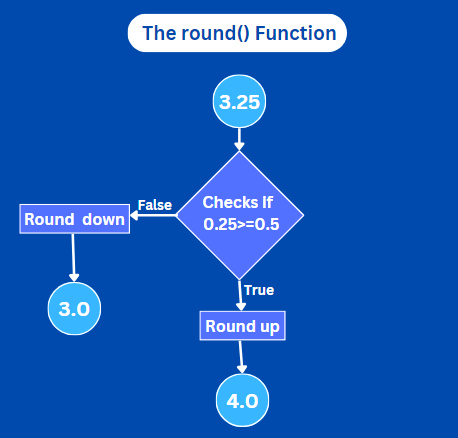
Let’s use this in the code:
import numpy as np
a_input = input("Enter the decimal numbers with separating spaces: ")
arr = np.array([float(x) for x in a_input.split()])
d_places = int(input("Enter the number of decimal places to round to: "))
rounded_arr = np.round(arr, d_places)
print("Original array:", arr)
print("Rounded array:", rounded_arr)
Working:
- It asks the user to enter decimal numbers separated by spaces.
- It takes the input, splits it by spaces, converts each part to a floating-point number, and creates an array from those numbers.
- Then, it asks the user how many decimal places to round to.
- It rounds each number in the array to the specified number of decimal places.
- Finally, it prints both the original array and the rounded array.
Output:
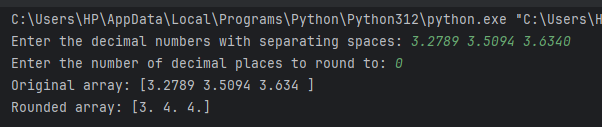
2. The floor() Function
The floor() is another function in the NumPy library which helps to round numbers down to the nearest whole number or integer.
The syntax form is like this:
np.floor(array)
Here,
- array is the input data.
How it works:
It always drops the decimal part and rounds to the lower whole number. In the case of positive numbers, it cuts off the decimal part and makes it the smaller whole number. For negative numbers, it moves towards zero and becomes the closest smaller whole number.
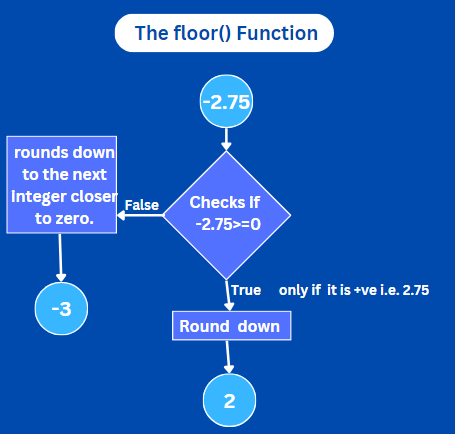
Let’s relate with code:
import numpy as np
a_input = input("Enter the decimal numbers with separating spaces: ")
arr = np.array([float(x) for x in a_input.split()])
rounded_arr = np.floor(arr)
print("Original array:", arr)
print("Rounded array:", rounded_arr)
This code does these:
- Asks for decimal numbers separated by spaces.
- Converts them into floating-point numbers and makes an array.
- Round down each number to the nearest whole number.
- Prints the original array and the rounded array.
Output:
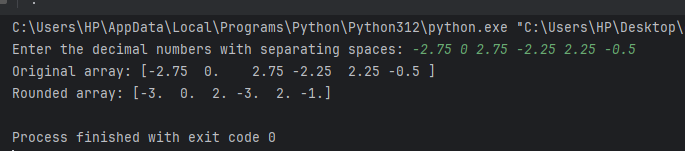
3. The ceil() Function
The ceil() function takes a number or an array of numbers as input and rounds them up to the nearest integer that is greater than or equal to the original number.
Its syntax is as follows:
np.ceil(array)
Here,
- array is the input data.
How it functions:
It always goes to the next higher whole number, ignoring the decimal part. For positive numbers, it rounds up. For negative numbers, it goes to the next integer farther from zero.
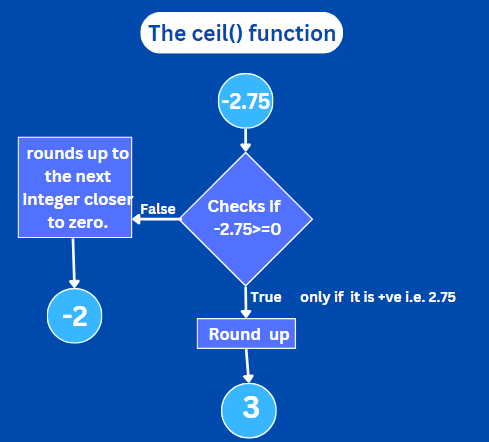
Let’s implement with a code:
import numpy as np
a_input = input("Enter the decimal numbers with separating spaces: ")
arr = np.array([float(x) for x in a_input.split()])
rounded_arr = np.ceil(arr)
print("Original array:", arr)
print("Rounded array:", rounded_arr)
The working of this code is similar to the above two, except it rounds up each number to the nearest whole number.
Output:
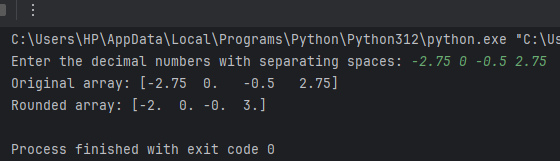
The ceil() and floor() functions can be quite confusing, right? Don’t worry, as we have a trick to help you get it right!
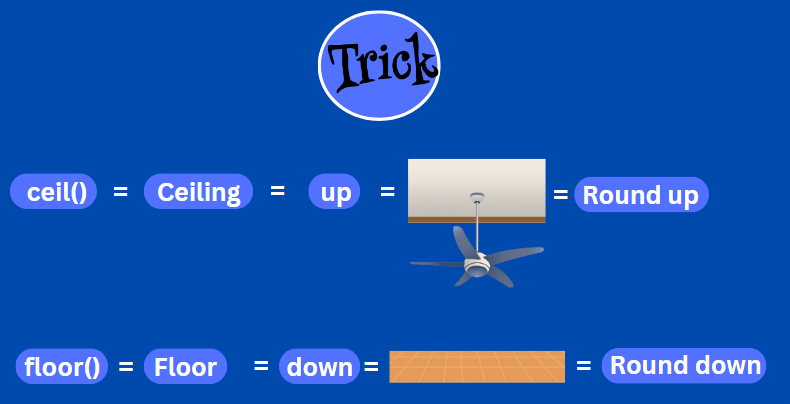
4. The trunc() Function
The trunc() function takes a number or a group of numbers and chops off the decimal part, leaving only the integer part.
The basic syntax is as follows with an array as the input data:
np.trunc(array)
How it performs:
It just cuts off the decimal part of the number without rounding. Always goes toward zero. For positives, it keeps the integer part. For negatives, it keeps the integer part and the negative sign.
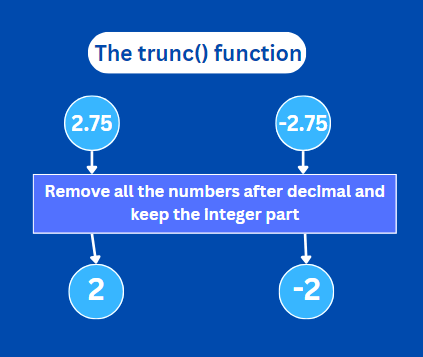
Let’s use this in the code:
import numpy as np
a_input = input("Enter the decimal numbers with separating spaces: ")
arr = np.array([float(x) for x in a_input.split()])
rounded_arr = np.trunc(arr)
print("Original array:", arr)
print("Rounded array:", rounded_arr)
This code takes decimal numbers as input, turns them into an array, and then removes the decimal part to keep only the integer part using trunc().
Output:
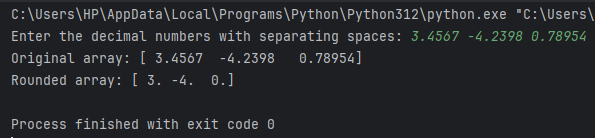
5. The around() Function
The around() rounds the input to a specified number of decimals. It’s like round() in Python but gives more control over how precise the rounding is.
The syntax for this is:
np.around(array, decimals)
Here,
- array is the input data,
- decimals are the specified limit to which it needs to be rounded.
How it works:
It rounds the input number to the nearest value with the specified number of decimals. If the decimal part is exactly halfway between two rounded values, around() rounds to the nearest even value for a more balanced approach to rounding.
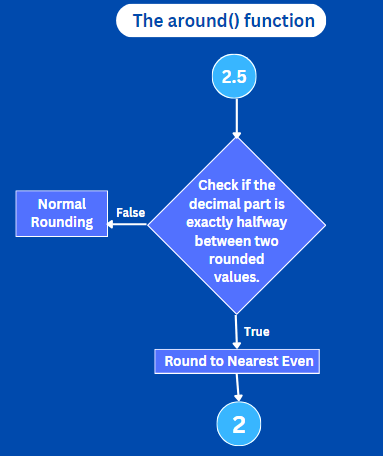
Let’s implement this in the code:
import numpy as np
a_input = input("Enter the decimal numbers with separating spaces: ")
arr = np.array([float(x) for x in a_input.split()])
rounded_arr = np.around(arr, 0)
print("Original array:", arr)
print("Rounded array:", rounded_arr)
This code takes decimal numbers as input, makes them into an array, and rounds each number to the nearest whole number using around().
Output:
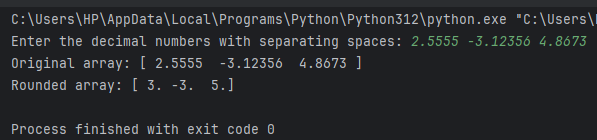
Summary
Making numbers simpler helps in math. In this article, we explored how to round off numbers in Python using NumPy. The round() function rounds to the nearest whole or specified decimal places, floor() rounds down, ceil() rounds up, trunc() chops off decimals, and around() offers precise rounding. Hope this makes rounding numbers clearer for you!
If you like this one, consider reading:
- Finding Max and Min Values Across Arrays in Python
- Find the Product of Array Elements in Python (3 Easy Ways)
Reference
https://numpy.org/doc/stable/reference/generated/numpy.round.html