Python’s NumPy library is very versatile. It has predefined functions for even finding maximum and minimum elements between two arrays. Let’s learn about them in this article. Here we will understand the fmax() and fmin() in detail, starting from their syntax to their use cases. Let’s start.
Why Find Max and Min Values?
Maximum is the highest value among a data set, and minimum is the lowest value of a data set. Calculating the maximum and minimum of a data set is important. When you have lots of numbers, finding the biggest and smallest helps you see how wide the range is.
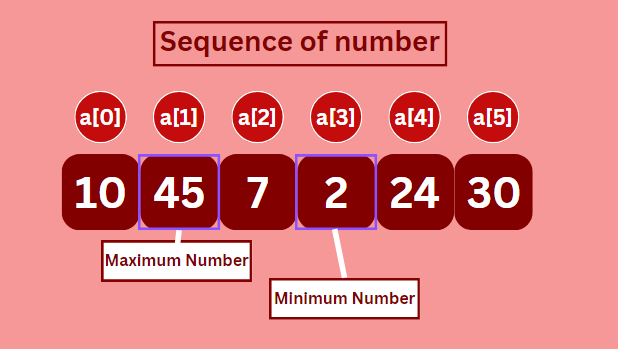
Sometimes, in programs, you need to do different things depending on the biggest or smallest number. So, finding them helps you make smart decisions in your code. If you’re expecting numbers to be within certain limits (like temperatures not going below freezing), checking against the maximum and minimum values helps catch mistakes or unexpected data.
NumPy fmax() Function
The fmax() function compares two arrays of numbers, element by element, and returns a new array containing the maximum values from each position in the original arrays.
The syntax of this function looks like:
numpy.fmax(array1, array2, /, out=None, *, where=True, casting='same_kind', order='K', dtype=None, subok=True[, signature, extobj])
Here,
- array1 and array2 are the lists of numbers whose elements will be compared one by one.
- out (optional): You can choose where to put the result. If you don’t say, it gives you a new array.
- where (optional): This is like a map telling where to copy numbers from the old arrays to the new ones. By default, it copies where it’s true.
- dtype (optional): It decides what type the new array should be. If you don’t say, it figures it out from the old arrays.
- Other parameters (casting, order, subok, etc.): These are extra settings you can adjust if you want, but usually, you don’t need to bother because they have sensible defaults.
How it works:
It compares two lists of numbers, element by element, and returns a new list containing the maximum value from each position in the original lists. For example, if you have two arrays: [3, 7, 5] and [2, 8, 6], fmax() will compare each pair of numbers: (3, 2), (7, 8), and (5, 6). It’ll pick the bigger number from each pair and give you back a new array: [3, 8, 6].
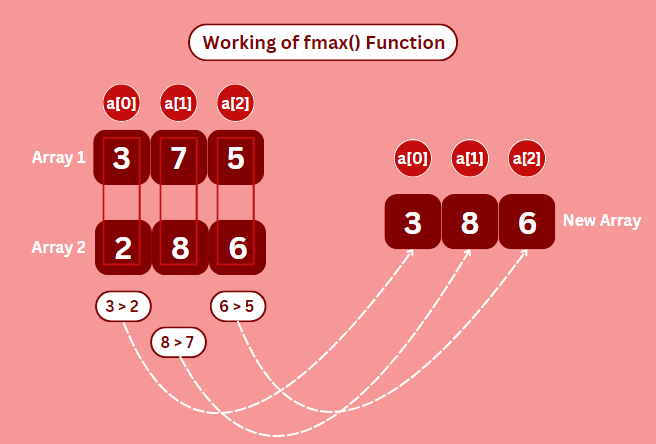
Let’s see its implementation in code:
import numpy as np
user_array1 = input("Enter the elements of first array : ")
user_array2 = input("Enter the elements of second array : ")
array1 = np.array([int(x) for x in user_array1.split()])
array2 = np.array([int(x) for x in user_array2.split()])
result = np.fmax(array1, array2)
print("Array containing the max numbers ", result)
This code will use fmax() to compare the two user-defined arrays, find the maximum elements, and print a new array containing those maximum numbers.
Output:
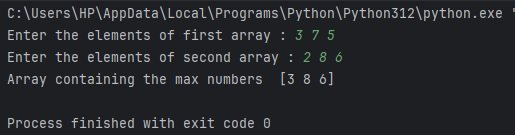
NumPy fmin() Function
The fmin() function compares two lists number by number. It gives you back a new list with the smallest numbers from each position in the original lists.
Here is what its syntax looks like:
numpy.fmin(x1, x2, /, out=None, *, where=True, casting='same_kind', order='K', dtype=None, subok=True[, signature, extobj])
How it works:
fmin() looks at two lists of numbers and picks out the smallest number from each matching position. For example, if you have two arrays: [4, 9, 1] and [7, 5, 3], fmin() will compare each pair of numbers: (4, 7), (9, 5), and (1, 3). It’ll pick the smaller number from each pair and give you back a new array: [4, 5, 1].
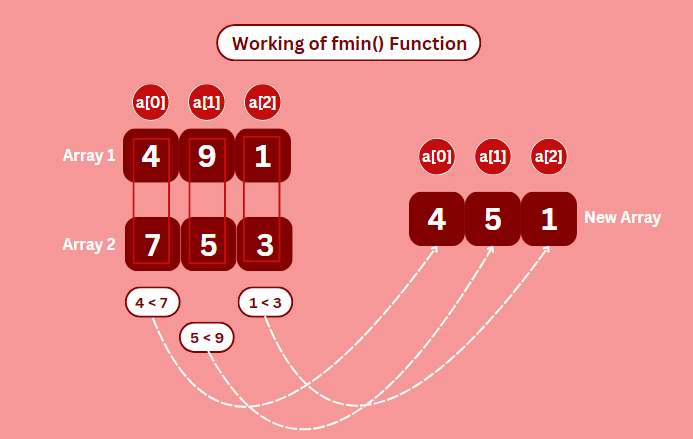
Let’s understand it with code:
import numpy as np
user_array1 = input("Enter the elements of first array : ")
user_array2 = input("Enter the elements of second array : ")
array1 = np.array([int(x) for x in user_array1.split()])
array2 = np.array([int(x) for x in user_array2.split()])
result = np.fmin(array1, array2)
print("Array containing the min numbers ", result)
This code will use fmin() to compare two arrays we make, find the smallest numbers, and show them in a new array.
Output:
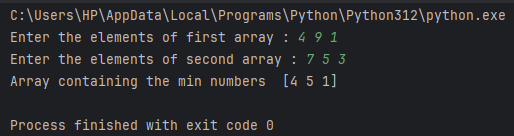
Summary
We have reached the bottom line. Here we discussed fmax() and fmin(), their syntax, working, and use cases. I hope that you have gone through each section thoroughly. And if you did, now you can easily calculate the array containing maximum or minimum elements by comparing any two arrays. Python offers more amazing libraries like Selenium and OpenCV, so don’t forget to check them out.
Reference
https://numpy.org/doc/stable/reference/generated/numpy.fmin.html