Exponential operations mean multiplying a number by itself several times. And if you want to do it for big numbers, it would be a very complex process. But don’t worry, we have NumPy to provide us with ease. The purpose of this article is to explain how we can use this tool to do all sorts of cool number crunching and math stuff in our computer programs.
An Introduction to Exponential Operations
When you do an exponential operation, you’re just taking a number and multiplying it by itself a bunch of times. For in 4^3, the 4 is the number we start with, and the 3 tells us how many times to multiply 4 by itself. So, 4^3 means we multiply 4 by itself 3 times: 4 x 4 x 4 = 64.
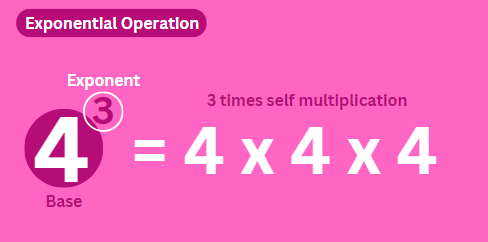
Exponential operations are quite important in science and engineering because they help us understand how things change over time. Like in physics, they help us describe things like how atoms break down, how populations grow, and even how electricity flows in circuits. In engineering, they’re used to figure out how things will grow or change in the future, like predicting how chemicals will react or how the environment might change over time.
Doing exponential operations efficiently can be tough, especially when dealing with really big numbers. It requires a lot of computing power and can slow things down if not done well. So, scientists and engineers are always looking for better ways to tackle these challenges. And one of those ways in programming is Python’s Numpy, let’s see how.
NumPy.power() in Python
NumPy is a Python library for numerical computing. It makes working with numbers way easier, especially when we have a bunch of them. Now, one cool thing NumPy can do is raise numbers to power. So, if we have a number and we want to multiply it by itself a certain number of times, NumPy’s power function can be used.
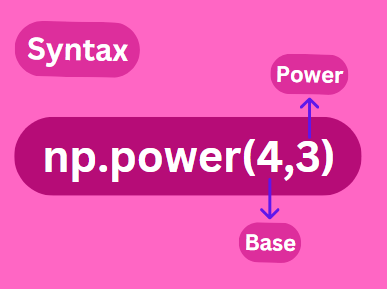
When we use NumPy’s power function, we write “np.power” followed by parentheses. Inside the parentheses, we put two numbers: the base number (the one we’re multiplying) and the exponent (how many times we’re multiplying it by itself). For example, “np.power(4, 3)” means we’re raising 4 to the power of 3, which equals 64.
Example Usage of NumPy.power() Function
Let’s see some examples to get more clear.
Example 1
Here we will use NumPy to calculate the result of raising a base number to a specified exponent entered by the user and then we’ll print the result.
import numpy as np
base = float(input("Enter the base number: "))
exponent = int(input("Enter the exponent: "))
result = np.power(base, exponent)
print("Result of", base, "raised to the power of", exponent, "is:", result)
Output:

Example 2
In this example, we will take numbers separated by spaces from the user. Then, we’ll convert them into a NumPy array and ask the user for an exponent. After that, we’ll raise each number in the array to that exponent and show both the original array and the result of raising each number to the given exponent.
import numpy as np
store = input("Enter numbers separated by spaces: ")
numbers = np.array([float(num) for num in store.split()])
exponent = float(input("Enter the exponent: "))
result = np.power(numbers, exponent)
print("Original array:", numbers)
print("Result of element-wise exponentiation (each number raised to the power of", exponent, "):", result)
Output:

Example 3
Let’s see if we can handle large datasets using this function. We will create a large list of random numbers between 1 and 100 using NumPy. Then, we’ll square each number in the list and display the first 10 original numbers along with their squared values.
import numpy as np
store = input("Enter numbers separated by spaces: ")
numbers = np.array([float(num) for num in store.split()])
exponent = float(input("Enter the exponent: "))
result = np.power(numbers, exponent)
print("Original array:", numbers)
print("Result of element-wise exponentiation (each number raised to the power of", exponent, "):", result)
Output:

Points to Remember
Here are five important things to remember when using NumPy’s power function:
- Data Types: Check that your input data and exponent have compatible types.
- Broadcasting: Understand how NumPy combines arrays of different shapes.
- Performance: Large data or high exponents may affect speed.
- Overflow/Underflow: Be cautious with very large or small numbers to avoid errors.
- Complex Numbers: NumPy’s power function works with complex numbers too.
Conclusion
In this article, we learned in detail about exponential operations and their significance. Additionally, we have seen how we can implement exponential operations in programming using NumPy’s power function. The examples I have used may have clarified your concepts even more. I hope you followed well and gained knowledge.
Feel free to check out more such NumPy blogs:
- Python NumPy divide() Function (With Examples)
- NumPy Array Addition with numpy.add() and Addition Operator
Reference
https://numpy.org/doc/stable/reference/generated/numpy.power.html