Working with image sizes can sometimes be very stressful. Did you know you can easily handle it with Python’s OpenCV library? In this article, we’ll dive deep into image sizes, learning how to obtain dimensions and resize images accurately without losing any data. But first, if you haven’t read the introduction to OpenCV, visit here to continue. Assuming you’re done, let’s begin!
Understanding Image Size
Image size in OpenCV means how big or small an image is. It’s about how many tiny dots called pixels make up the image, both in width and height. Knowing this is important when you’re doing stuff with images like making them bigger or smaller, cropping, scaling, or applying various transformations.
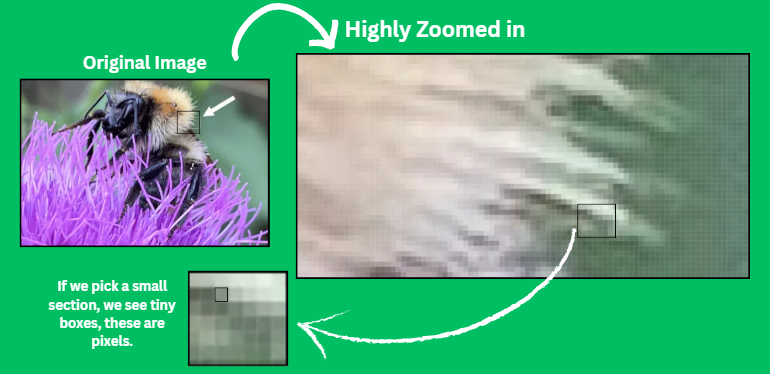
If you’re using Python, OpenCV has special tools to help you do all these things with images with ease. In OpenCV, pictures are like tables made up of numbers, called NumPy arrays. These tables have different sizes depending on whether the picture is black and white or color.
- For black and white pictures, the table has two measurements: how tall and wide it is. Sometimes, grayscale pictures can be saved in a way that’s a bit confusing. Even though they’re supposed to have only one color channel, they might still be stored as if they have three channels, with all channels having the same color info. This can happen because of how the pictures are changed or saved.
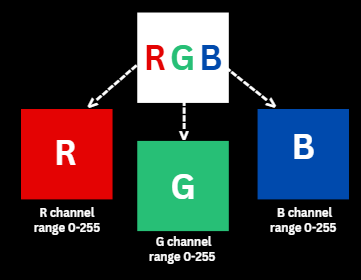
- But for color pictures, it’s a bit more complex. The table has three measurements: how tall and wide it is, and also how many colors are mixed together, usually three, which stand for red, green, and blue.
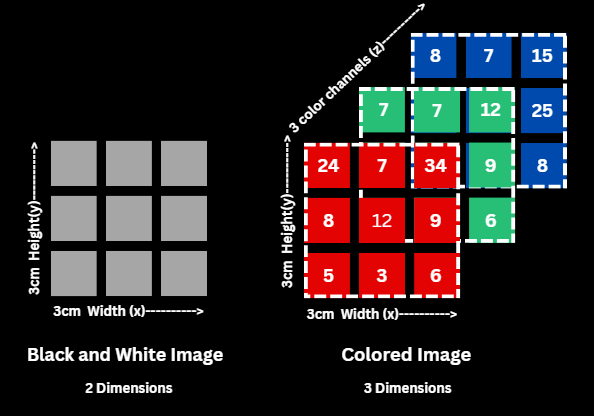
Accessing Image Size Using cv2.imread()
Let’s see how we can fetch the image size and display dimensions using OpenCV. We will use the given eye softening image of a kitten for processing.
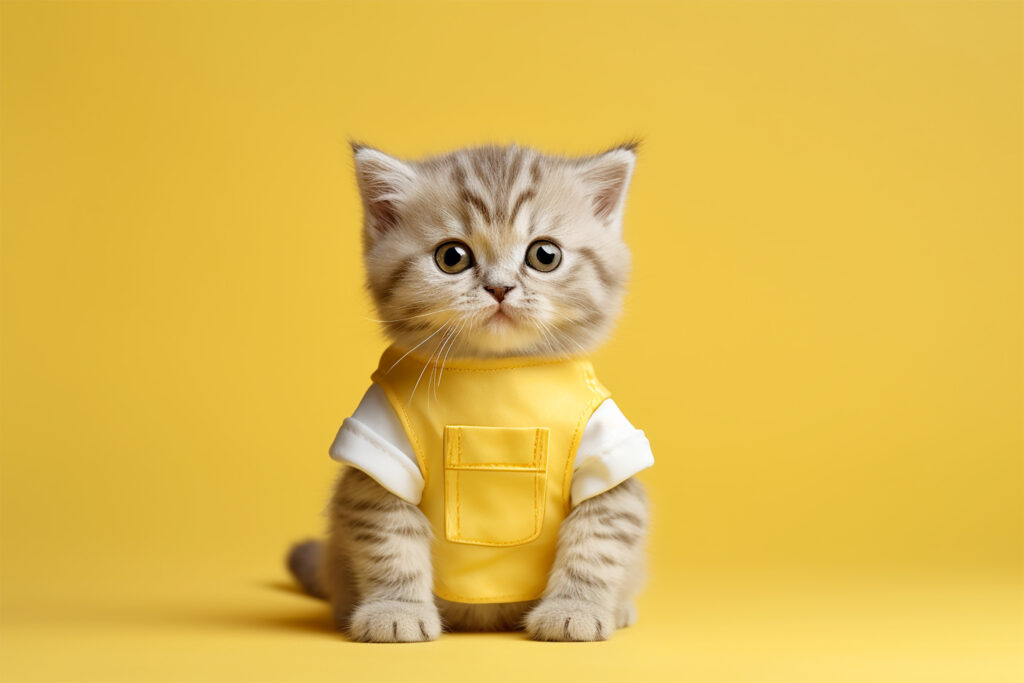
Example 1
You can find out how big an image is by using the “.shape” with the NumPy array that holds the image. When you do that, it gives you a set of numbers in brackets, like this: (height, width, channels).
import cv2
img = cv2.imread('color.jpg')
Size = img.shape
print("Dimension of the image are :", Size)
This code prints the dimensions (height, width, and number of color channels) of the ‘color.jpg‘ image.
Output
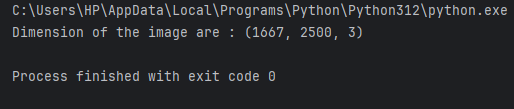
Example 2
We can also access each member of the size array separately by using array indexing like this:
import cv2
img = cv2.imread('color.jpg')
print("Height :", img.shape[0], "px")
print("Width :", img.shape[1], "px")
print("Number of Channels : ", img.shape[2])
This code prints all the dimensions separately.
Output

Resizing Images Using cv2.resize()
When you resize images, you’re basically changing their size. OpenCV has this thing called cv2.resize() to help with that.
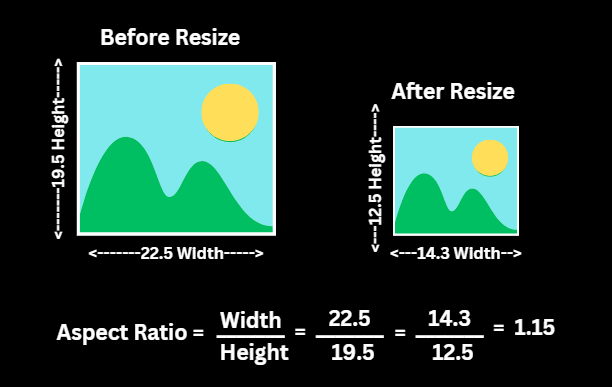
When you resize, it’s nice to keep the picture looking the same, so you don’t squish or stretch it weirdly. You can figure out the aspect ratio by doing width divided by height. Then, if you want to resize and keep the aspect ratio, you can calculate the new size using one of the dimensions and the aspect ratio.
Example
Let’s try to resize the “color.jpg“.
import cv2
img = cv2.imread('color.jpg')
width = img.shape[1]
height = img.shape[0]
aspect_ratio = width / height
new_height = int(input("Enter the new height of the image: "))
new_width = int(new_height * aspect_ratio)
resized_image = cv2.resize(img, (new_width, new_height))
cv2.imwrite("Resize.jpg", resized_image)
print("Image resized successfully")
This code reads an image and asks the user for a new height. Then, it calculates the width based on the aspect ratio, resizes the image accordingly, saves it as ‘Resize.jpg’, and prints a success message.
Output
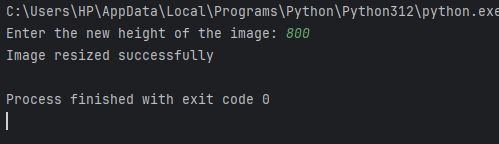
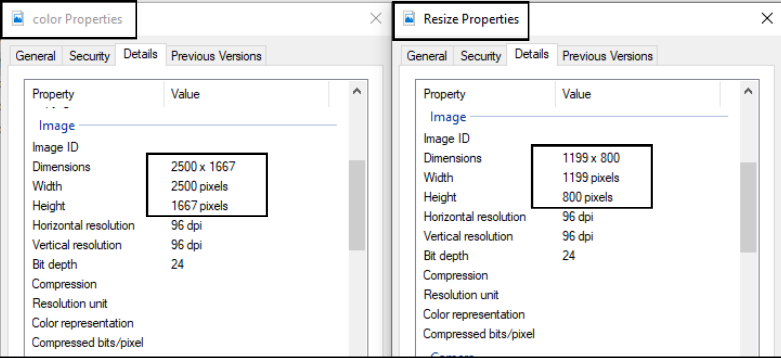
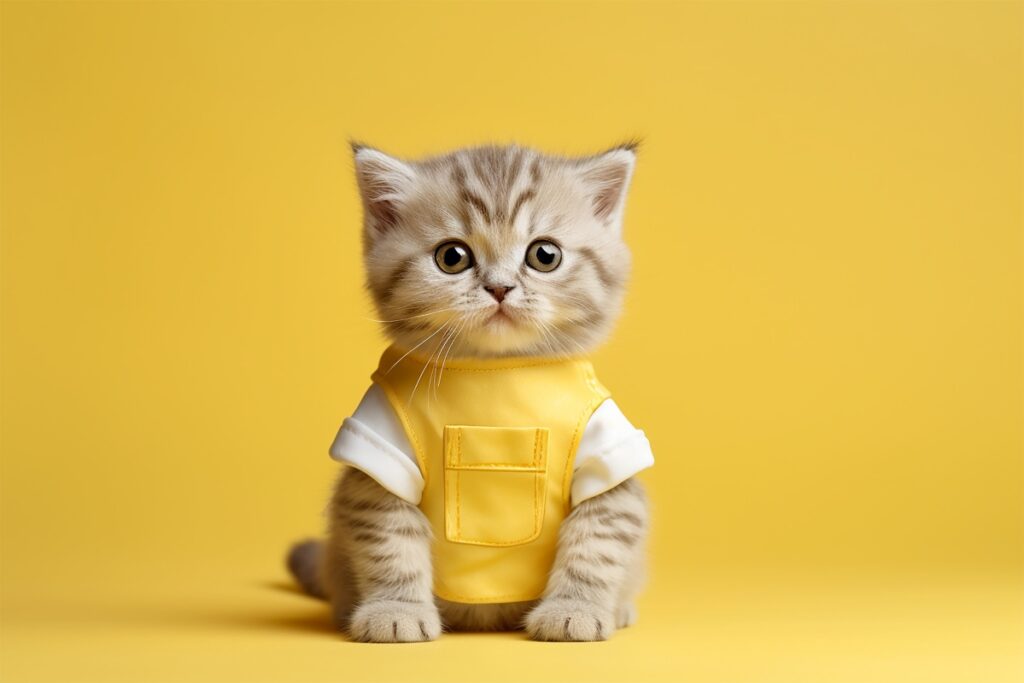
Conclusion
Did you enjoy this article? It was fun, wasn’t it? We got to learn about so many new things. We got into in-depth concepts about images, including their dimensions, pixels, height, width, and RGB channels. We also learned how to retrieve this information using Python programming. Additionally, we explored aspect ratio and how to resize an image. Did you know that with OpenCV, you can write text on images? Don’t believe me? Check it out yourself!
Reference
https://stackoverflow.com/questions/19098104/python-opencv2-cv2-wrapper-to-get-image-size