Hello Python lovers! Web applications get new stuff added to them every couple of weeks to keep users interested. But to make sure all these new things work properly and the website still looks good, we need to do something called automation testing.
In this article, we will introduce you to a web-testing library in Python. You will be amazed to learn about Selenium and its features and will realize why it is loved by developers so much. Let’s begin.
Introduction to Selenium
Selenium is a very popular open-source tool used for test automation on web browsers. Jason Huggins created Selenium in 2004.
To begin using Selenium with Python, your first task is to write functional test cases using the Selenium web driver. After that, you’ll send a request to the Selenium server, which works behind the scenes. This server will then automatically run your test cases on the web browsers. Test scripts can be created in various programming languages like Java, Python, C#, etc.
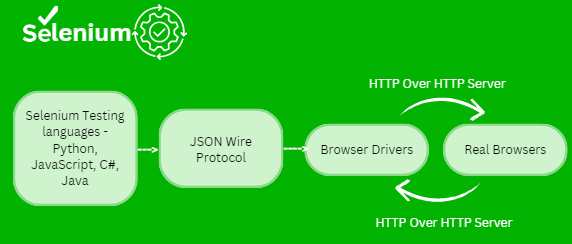
Selenium only allows the testing of web applications. It is a suite of four tools, each serving different testing needs. Let’s discuss a bit about each of them.
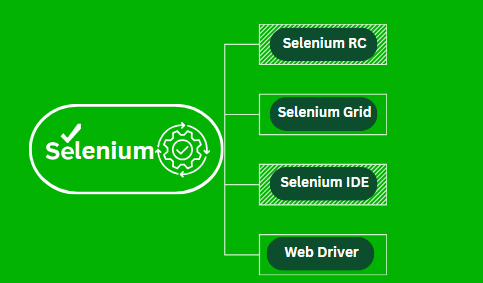
- Selenium RC (Remote Control) lets you write automated web applications using different programming languages. It helps deploy tests across multiple environments.
- Selenium Grid allows tests to run at the same time on many computers. It sends instructions to remote web browser instances. One computer acts as the main hub, controlling the tests.
- Selenium IDE doesn’t need any extra setup. Just install it as an extension in your browser. It helps make debugging tests easier by letting you set breakpoints and pause exceptions.
- Selenium Web Drivers are super important in the Selenium toolkit. They help test websites on different browsers, making sure they work well for everyone. These drivers work on different systems like Windows, Mac, Linux, Unix, and more.
Why Do We Use Selenium?
Selenium is the best choice because of the following features:
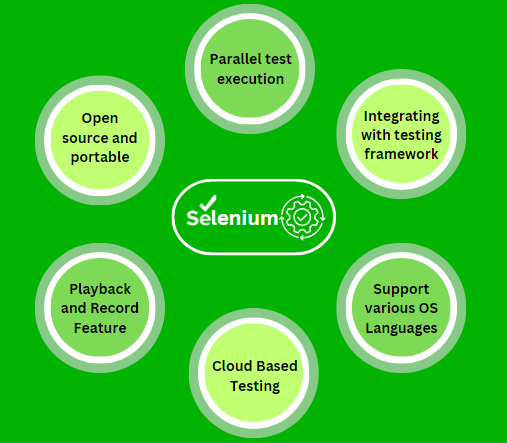
- Selenium is open-source and portable.
- It can run tests at the same time on different machines.
- It works smoothly with different testing frameworks.
- This can work on different types of computers and in many OS languages.
- It can perform tests on remote servers using cloud-based testing platforms.
- This can record and replay user interactions with web applications.
Installation and Setup of Selenium
To get Selenium on your computer, just type “pip install selenium” in your command prompt or terminal. This will download and install Selenium for you.
pip install selenium
Once you have installed Selenium, it gives you the tools you need. But to make it work with your specific web browser, you’ll need to get some extra drivers. Below are the download links for these drivers:
That’s it! Now you’re ready to use Selenium in your Python projects.
Getting Started with Selenium for Web Automation
Now that we have this library installed, let’s try to run a test code to learn how we can open any URL in the browser we want and test things in it.
Code:
from selenium import webdriver
import time
browser = webdriver.Chrome()
browser.get('https://codeforgeek.com/')
print(browser.title)
time.sleep(10)
browser.quit()
Working:
- The given code imports selenium and time library.
- It initiates a new instance of Chrome and navigates to the URL “https://codeforgeek.com/” using the .get() method.
- Then retrieves and prints the title of the webpage.
- It then pauses execution for 10 seconds using the time.sleep() function, allowing time for observation or for page elements to load.
- Finally, it closes the browser using the browser.quit().
Output:
The code opens a webpage, prints its title, waits for 10 seconds, and then closes the browser.
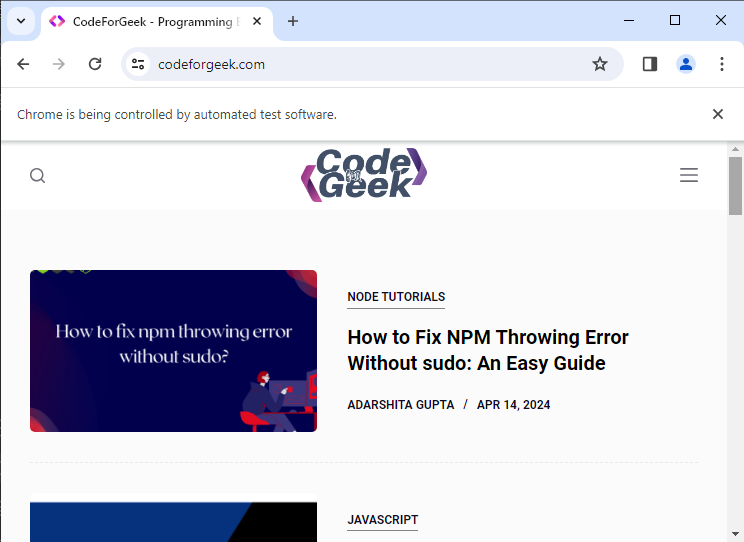
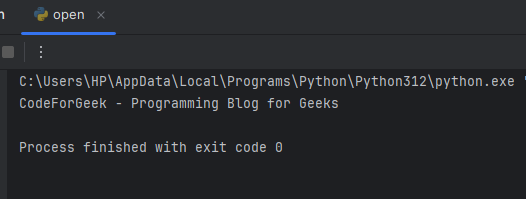
Summary
And we have reached the bottom. I hope you are clear with all the above mentioned concepts. We have learned about the upper layer of Selenium, its four main suites, along with its best features and installation process. We have also seen a simple program to clarify the workings of Selenium. We hope you enjoyed it.
Continue Reading:
Reference
https://www.selenium.dev/documentation/webdriver/getting_started