In this guide, we will walk you through the process of creating your own YouTube video downloader using Tkinter. With the video-sharing website’s immense popularity, having a simple and convenient solution for downloading your favourite videos can be a real game-changer. We will explore the basics of Tkinter and how it can assist in creating graphical user interface (GUI) applications such as a YouTube video downloader with ease.
Introduction to Tkinter
Tkinter is a Python library used in building graphical user interfaces (GUIs) for user interaction with programs. It offers ready-made widgets to easily create and organize components like buttons and text boxes.
Additionally, Tkinter simplifies event handling, enabling developers to define functions triggered by user actions, and enhancing the control and responsiveness of Python programs.
Execute the below command to install Tkinter on your system:
pip install tk
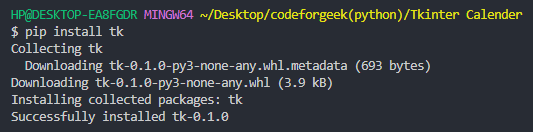
What is a YouTube Video Downloader?
A YouTube video downloader is a tool that lets users save videos from YouTube to their devices like computers, smartphones, or tablets. This tool allows offline viewing by downloading videos, even though YouTube’s terms usually don’t allow it without explicit permission due to copyright concerns.
The application that we are designing will require two inputs: the video URL and the location path where the video needs to be stored. Using the Pytube library, the video details will be fetched and the highest resolution stream will be identified. When the user clicks on the “Download” button, the Pytube library will be used to download the video. The status label will update to indicate the completion of the download or any relevant messages that may be necessary.
Creating YouTube Video Downloader Using Python
Now that we know what is a YouTube video downloader and how it works, let’s try to create it using Python code.
Step 1: Environment Setup
Let’s create a Python file since we are working with Python. Besides Tkinter, we also need to install “pytuber” library for downloading YouTube videos.
pip install pytube
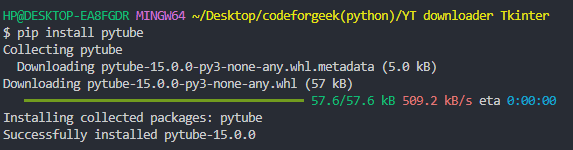
Step 2: Importing Modules
We need to import the necessary libraries like tkinter for the GUI and YouTube from pytube for YouTube video downloading.
import tkinter as tk
from pytube import YouTube
Step 3: Creating Application Window
Then we will create the main Tkinter window with a title and set the background color to black.
app = tk.Tk()
app.title("YouTube Video Downloader")
app.configure(bg="black")
Step 4: Creating and Placing Widgets
Next, we will create and customize various widgets such as label, entry, button, and status label. These widgets are packed into the main window with specific configurations for appearance and layout.
UrlLabel = tk.Label(app, text="Enter YouTube URL:", bg="red",fg="white", font=("Arial", 12, "bold"))
UrlLabel.pack(pady=10)
entry = tk.Entry(app, width=30, font=("Arial", 10))
entry.pack(pady=10)
PathLabel = tk.Label(app, text="Enter download path:", bg="blue",fg="white", font=("Arial", 12, "bold"))
PathLabel.pack(pady=5)
PathEntry = tk.Entry(app, width=30, font=("Arial", 10))
PathEntry.pack(pady=10)
Dbutton = tk.Button(app, text="Download Video", command=VideoDownload, bg="green", fg="white", font=("Arial", 12, "bold"))
Dbutton.pack(pady=10)
StatusLabel = tk.Label(app, text="", bg="black", fg="white", font=("Arial", 12, "bold"))
StatusLabel.pack(pady=10)
Step 5: Starting Event Loop
Next, we will write code to start the Tkinter main event loop to display the GUI and handle user interactions.
app.mainloop()
Step 6: Creating Video Download Function
def VideoDownload():
try:
url = entry.get()
youtube = YouTube(url)
video = youtube.streams.filter(progressive=True, file_extension="mp4").first()
PathDownload = PathEntry.get()
video.download(PathDownload)
StatusLabel.config(text="Download complete!")
except Exception as e:
StatusLabel.config(text="Error: " + str(e))
The VideoDownload function is triggered by pressing the “Download Video” button. It attempts to download the YouTube video indicated by the user-entered URL using the pytube library. The function accesses YouTube video streams and opts for the initial progressive stream with an MP4 extension. The download path is derived from the user’s input in the PathEntry widget. Upon successful download, the status label is updated to “Download complete!“. In case of an error, an appropriate error message is displayed.
Complete Code for Creating YouTube Video Downloader
import tkinter as tk
from pytube import YouTube
def VideoDownload():
try:
url = entry.get()
youtube = YouTube(url)
video = youtube.streams.filter(progressive=True, file_extension="mp4").first()
PathDownload = PathEntry.get()
video.download(PathDownload)
StatusLabel.config(text="Download complete!")
except Exception as e:
StatusLabel.config(text="Error: " + str(e))
app = tk.Tk()
app.title("YouTube Video Downloader")
app.configure(bg="black")
UrlLabel = tk.Label(app, text="Enter YouTube URL:", bg="red", fg="white", font=("Arial", 12, "bold"))
UrlLabel.pack(pady=10)
entry = tk.Entry(app, width=30, font=("Arial", 10))
entry.pack(pady=10)
PathLabel = tk.Label(app, text="Enter download path:", bg="blue", fg="white", font=("Arial", 12, "bold"))
PathLabel.pack(pady=5)
PathEntry = tk.Entry(app, width=30, font=("Arial", 10))
PathEntry.pack(pady=10)
Dbutton = tk.Button(app, text="Download Video", command=VideoDownload, bg="green", fg="white", font=("Arial", 12, "bold"))
Dbutton.pack(pady=10)
StatusLabel = tk.Label(app, text="", bg="black", fg="white", font=("Arial", 12, "bold"))
StatusLabel.pack(pady=10)
app.mainloop()
Output:
When we run the above code, a window like the following is displayed:
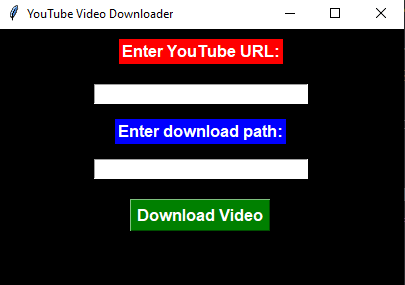
In the two input spaces provided, we need to fill in the URL of the desired YouTube video and the path location where we want the video to be downloaded:
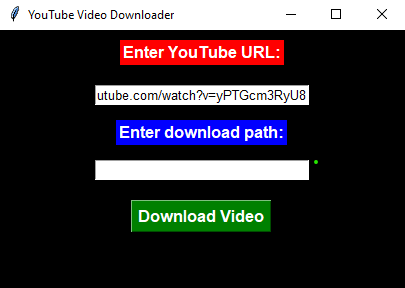
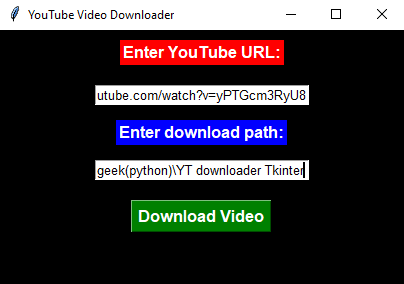
After clicking the download button, the download process starts and a success message is displayed upon completion:
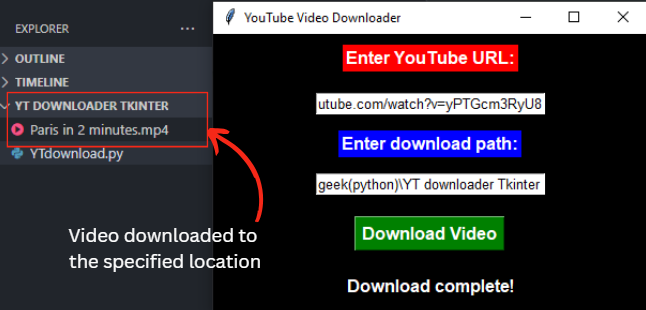
Conclusion
This is the end of this article. This guide has provided a step-by-step tutorial on how to create a YouTube video downloader using Tkinter in Python. Considering the immense popularity of YouTube, such a tool can prove to be quite useful. We have covered the basics of Tkinter, its installation and walked through the process of its implementation. By creating practical applications like this one, you can definitely upgrade your Python skills.
If you had fun reading this article, learn to make more such tkinter applications with the following listed articles –
Reference
https://stackoverflow.com/questions/76639341/python-youtube-video-downloader-using-link