One key aspect influencing the performance of network applications in Node.js is the TCP_NODELAY option. TCP_NODELAY is a key setting for improving data transmission in TCP connections. In the context of Node.js, we will explore its importance and learn how to use it to optimize data transfer. Through a straightforward program, we will demonstrate the real-world impact of turning TCP_NODELAY on or off. This will help developers understand how to use this option effectively to improve the performance and responsiveness of their network applications.
Overview of TCP
Transmission Control Protocol (TCP) is a fundamental communication protocol within the Internet Protocol (IP) suite. Operating at the transport layer, TCP provides reliable, connection-oriented communication between devices over a network. It ensures accurate and ordered delivery of data, making it suitable for applications where data integrity is crucial.
TCP makes sure information gets reliably from one place to another using tricks like saying “got it” (acknowledgements), controlling the flow of data, and checking for mistakes. It’s like making a secure call before sharing information, keeping the line open during the whole talk, and making sure the info arrives in the right order.
Overview of TCP_NODELAY
In Node.js and network apps, TCP_NODELAY is like a special setting for making data move faster in TCP connections. Turning on TCP_NODELAY means we are skipping a delay system (Nagle’s Algorithm), so information can be sent without waiting.
This is really important when we need information to travel quickly, like in apps where instant communication matters, such as real-time applications and interactive systems. Making network communication work well in Node.js apps is super important for making everything run better and handling more tasks. When the network is efficient, it not only makes things faster for users but also uses resources better, saving costs.
Significance of TCP_NODELAY:
- Turning off Nagle’s Algorithm with TCP_NODELAY makes a positive impact on how fast small packets of data are sent, reducing the delay. This is especially helpful for applications that need speedy and responsive communication.
- TCP_NODELAY is really useful in apps where things need to happen instantly, like online games, watching videos, and live chat. It is especially important in these cases because it ensures a smooth user experience by keeping the delay as low as possible.
- Developers can decide whether to use or not use TCP_NODELAY, depending on what their apps need. This gives them the ability to adjust how data is sent, customizing it to fit their application’s requirements.
Understanding Nagle’s Algorithm
Nagle’s Algorithm works by holding onto small pieces of data for a short time. It waits to see if more data is coming. If more data shows up or a timeout happens, it sends all the gathered data as a bigger packet. If no more data comes, it sends what it has after waiting for a bit
Picture it in a way that you have details about four fruits—apples, oranges, bananas, and grapes—and you want to send this info from one place to another. Think of each fruit as a tiny piece of data, and the basket as a packet that goes through the network.
Without Nagle’s Algorithm:
If we don’t apply Nagle’s Algorithm, you might send each fruit as soon as you have it. For example, you send an apple, then an orange, followed by a banana, and finally, grapes. Each fruit is sent separately.
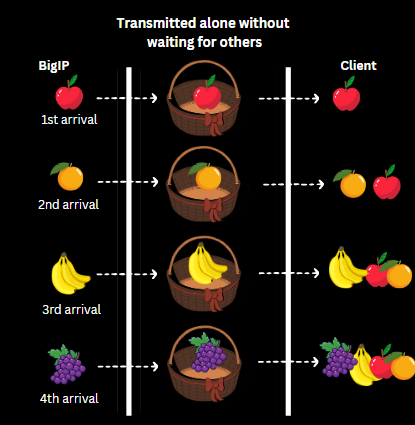
With Nagle’s Algorithm:
Now, let’s apply Nagle’s Algorithm to our fruit basket analogy. Instead of sending each fruit immediately, we wait a short period to see if more fruits are coming. During this time, we collect the fruits in a basket. If, within the waiting period, more fruits arrive, we group them together in the basket before sending them.
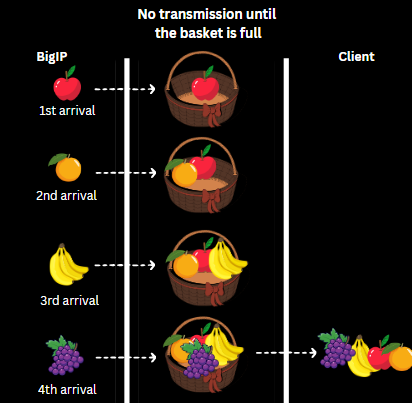
Why Disable Nagle’s Algorithm
It is necessary to keep in mind, depending on what you are doing, that sometimes the delay from Nagle’s Algorithm might be a problem. Especially in apps where fast communication is super important. In these cases, developers can turn off Nagle’s Algorithm using the TCP_NODELAY option to make sure data gets sent right away, some of the more reasons why we should disable Nagle’s Algorithm are:
- Nagle’s Algorithm, by intentionally delaying data, can increase latency, affecting user experience in real-time applications like online gaming or live streaming.
- Nagle’s Algorithm might send bigger packets than needed for apps with lots of small data, causing inefficiency and suboptimal use of network resources.
- For quick communication in scenarios like interactive systems or instant messaging, it’s necessary to turn off Nagle’s Algorithm using TCP_NODELAY for prioritizing low-latency transmission.
The setNoDelay() Method
In Node.js, the setNoDelay() function, is part of the Net module and Socket class. It is used to enable or disable the Nagle’s Algorithm.
Syntax:
socket.setNoDelay([noDelay])
Parameters: noDelay (optional boolean), if provided, sets the TCP NoDelay option to the specified boolean value. If you want to check the current setting, you can call socket.setNoDelay() without passing any arguments, and it will return the current NoDelay setting.
When the TCP NoDelay option is enabled (socket.setNoDelay(true)), it means that the socket will try to send data as soon as it is available, without waiting to accumulate a large amount of data. This can be beneficial for applications that require low latency, such as real-time communication systems.
When TCP NoDelay is turned off (socket.setNoDelay(false)), Nagle’s Algorithm is used. This algorithm groups small messages to decrease network packet count, efficient for large data transfers. However, it introduces latency, making it less suitable for real-time applications.
Disabling Nagle’s Algorithm by Enabling TCP_NODELAY
To control Nagle’s Algorithm in Node.js, you can use the setNoDelay method on a net.Socket object. Here’s a real-life example of a basic Node.js program to show how TCP_NODELAY works. It creates a server and a client. The server echoes back data from the client, and we use setNoDelay to turn off Nagle’s Algorithm on the client side.
server.js:
// server.js
const net = require('net');
const server = net.createServer((socket) => {
socket.on('data', (data) => {
console.log(`Received data from client : ${data}`);
socket.write(`Server got the confirmation: ${data}`);
});
});
const PORT = 3000;
server.listen(PORT, () => {
console.log(`Server listening on port ${PORT}`);
});
client.js:
// client.js
const net = require('net');
const client = net.createConnection({ port: 3000 }, () => {
console.log('Connected to server');
// Set TCP_NODELAY option to disable Nagle algorithm
client.setNoDelay(true);
client.write('Hello, I have recieved all the fruits!');
});
client.on('data', (data) => {
console.log(`Received from server: ${data}`);
client.end();
});
client.on('end', () => {
console.log('Bill paid and connection ended');
});
Working:
- Server (server.js): Creates a TCP server using the net module. Listens for incoming connections on port 3000. Upon receiving data from a client, logs the received data, and sends a confirmation message back to the client.
- Client (client.js): Creates a TCP connection to the server on port 3000. Sets the TCP_NODELAY option to disable the Nagle algorithm, aiming for quicker data transmission. Write a message (“Hello, I have received all the fruits!”) to the server. Listens for data from the server, logs it, and then ends the connection.
In the client script (client.js), by using client.setNoDelay(true);, Nagle’s Algorithm is turned off. This action reduces delays in data transmission, prioritizing immediate data transfer.
Output:

You can run the server in one terminal window (node server.js) and the client in another (node client.js). You should observe the server echoing back the data received from the client.
Summary
Now that we have come to an end, we hope that you are clear with the use of setNoDelay(), the idea of TCP_NODELAY, Nagle’s Algorithm, and the need to remove it. When using TCP sockets in a Node.js application, we must ensure it is quick and efficient, especially in a real-time conversation application. This is why we can remove algorithms like Nagle’s Algorithm using the above-described method to ensure speed and a nice user experience.
Continue Reading –
- https://codeforgeek.com/inter-process-communication-in-nodejs/
- https://codeforgeek.com/node-sass-in-nodejs/
Reference
https://github.com/nodejs/node/issues/34185