Have you ever heard about the node-sass library? As web projects become more intricate, using efficient stylesheets is crucial. CSS pre-processors, such as Sass, are helpful in this context. Sass is like an improved version of regular stylesheets, providing advanced features and enhancing organization for easier management. The cool part is that we can use Sass with the help of a tool called node-sass which is a part of the NPM repository. In this article, we will be taking a closer look at node-sass and how it helps us to style our websites elegantly.
What is node-sass?
Sass stands for Syntactically Awesome Stylesheets. It is a language extension for stylesheets that adds features such as variables, nesting and mixins, making CSS easier to write and maintain. Node-sass acts as a helpful assistant for web developers. It’s a tool that enables the use of Sass in Node.js projects. Picture it as a translator that takes the advanced features of Sass, and makes them usable for our websites. Its goal is to simplify and improve the styling process for websites.
Now, LibSass is like the brain behind node-sass. Node-sass is a wrapper around LibSass, which is written in C++. Since Node.js understands JavaScript and LibSass is in C++, node-sass acts as a translator between them. It provides a Node. js-friendly interface to interact with LibSass and integrate LibSass into Node.js projects. This helps us utilize Sass for styling web applications.
Working of node-sass
Let’s discuss the actual work that node-sass does. When this package is installed in your project, you import “node-sass” into your main server JavaScript file. In the package.json of your project, we add an instruction line “compile-sass” into the scripts section, like this: “<location of the sass file> -o <location where you need to store the CSS file>”. When we run the npm run command, our sass file is translated into a normal CSS file, which can be easily understood by our Node.js.
Key Features of node-sass
Node-sass, a widely used library for incorporating Sass into Node.js projects, provides numerous essential features and benefits. Some of them are listed below:
- Node-sass makes your website style faster by quickly converting your Sass code into CSS, resulting in faster loading and easy changes.
- Node-sass CLI converts Sass/SCSS to CSS in the terminal, which is handy for automating build processes.
- Node-sass remembers previous translations and utilizes a cache to save time and effort during compilation.
- In large projects, organization matters. Node-sass makes it well-structured as it breaks it into smaller files, Also it uses features like partials and imports wisely as it keeps everything tidy.
- Node-sass creates source maps that aid in debugging. These maps link the compiled CSS back to the original Sass or SCSS source code, helping developers identify and resolve issues.
- It works smoothly on Windows, macOS, and Linux systems without any compatibility concerns for developers.
Installation of node-sass
Now, before using sass in our project files, we need to install its package and also set some basic configurations. NPM or the Node Package Manage helps to manage dependencies and packages proficiently.
In our project directory, we open the command prompt and type the following command to install node-sass:
npm install node-sass --save-dev
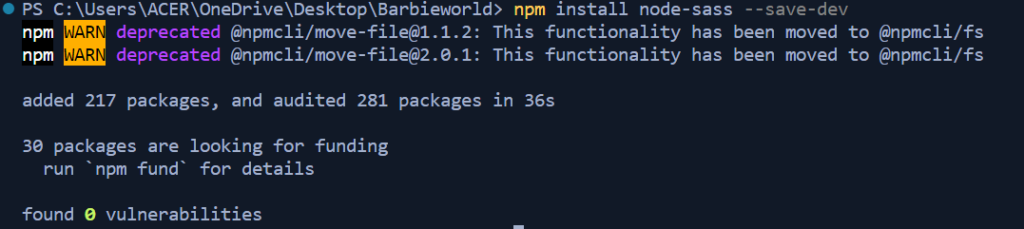
The above command installs the “node-sass” package as a development dependency for our Node.js project.
Integrate node-sass with Node.js
Since node-sass is installed, let’s explore how to integrate and configure it into a real-life application. With the widespread popularity of Barbie, suppose we are tasked with developing a Barbie game project. For any game project, a crucial component is a well-designed login page. Let’s create an elegant login page for the game using Sass, and employ Node-sass to facilitate the integration of Sass into Node.
The file structure for our project is given below:
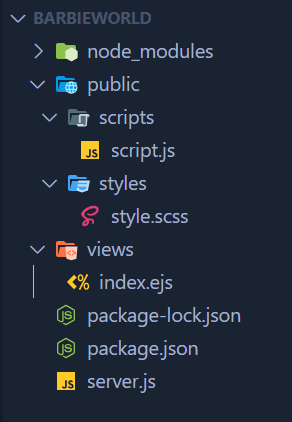
We have created an index.ejs file to contain the structure of our webpage with the following content:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="/styles/style.css">
<title>Barbie World</title>
</head>
<body>
<div class="login-container">
<h1>Barbie World</h1>
<form id="login-form">
<label for="username">Username:</label>
<input type="text" id="username" name="username" required>
<label for="password">Password:</label>
<input type="password" id="password" name="password" required>
<button type="submit">Login</button>
</form>
</div>
</body>
</html>
To enhance our login page, we have created a style.scss file to house the Sass styling code for our webpage, with the following content:
$white: #ffffff;
$baby-pink: #ffb6c1;
$fuchsia-pink: #ff00ff;
body {
margin: 0;
padding: 0;
height: 100vh;
display: flex;
align-items: center;
justify-content: center;
background: linear-gradient(45deg, $white, $baby-pink, $fuchsia-pink, $baby-pink, $white);
background-size: 400% 400%;
animation: gradientMotion 15s ease infinite;
}
.login-container {
text-align: center;
background-color: rgba($white, 0.8);
padding: 20px;
border-radius: 10px;
}
h1 {
color: $fuchsia-pink;
}
form {
display: flex;
flex-direction: column;
align-items: center;
margin-top: 20px;
label {
margin-bottom: 5px;
}
input {
padding: 8px;
margin-bottom: 15px;
border: 1px solid $fuchsia-pink;
border-radius: 5px;
width: 200px;
}
button {
padding: 10px 20px;
background-color: $fuchsia-pink;
color: $white;
border: none;
border-radius: 5px;
cursor: pointer;
}
}
@keyframes gradientMotion {
0% {
background-position: 0% 50%;
}
50% {
background-position: 100% 50%;
}
100% {
background-position: 0% 50%;
}
}
The script.js file has the logic for operating the login page. Using the “npm init -y“, we create a package.json file for the project. Also, we install other important packages like ejs and Express. Also, we have a server.js file for the server management of our project with the following content:
const express = require('express');
const path = require('path');
const app = express();
const port = 3000;
app.set('view engine', 'ejs');
app.use('/styles', express.static(path.join(__dirname, 'public', 'styles')));
app.use(express.static(path.join(__dirname, 'public')));
app.get('/', (req, res) => {
res.render('index');
});
app.listen(port, () => {
console.log(`Server is running at http://localhost:${port}`);
});
This code will make our server run on localhost, and the login page will appear there.
Configuring node-sass
We have a Sass file that needs to be converted into a CSS file understandable by our Node environment. We need to configure it in such a way that the content in the file “style.scss” is converted to CSS content and stored in a newly created file named “style.css”.
For this, we add an instruction line “compile-sass” into the scripts section of our package.json file, like the one given below:

“public/styles/style.scss” is the directory to our sass file and “public/styles” is the location where we need to create the style.css file to store the converted content. Now to function this process we write the following command:
npm run compile-sass

This command will successfully convert Sass to CSS.
We will see an update in our file structure as a new file is added to the styles folder:
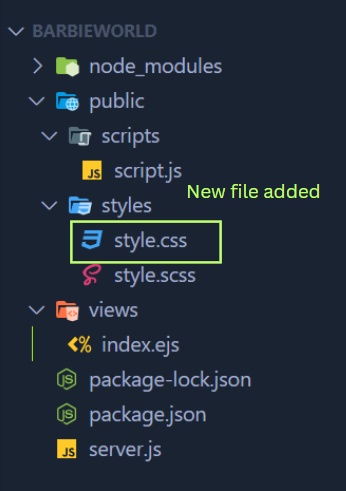
Now, if we run our project using:
node server.js
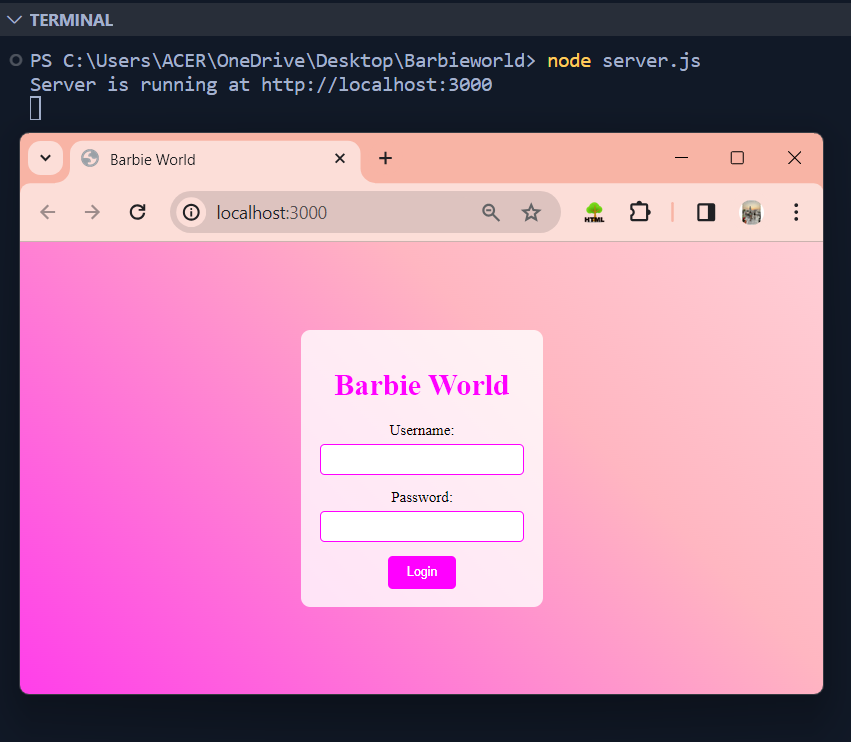
We see that our login page is hosted successfully and the Sass styling code is working perfectly.
Compatible node-sass Versions for Node.js
To work smoothly without any hurdles and bugs, it is very important that you use the correct version of node-sass with Node.js. You have to make sure that they are compatible with each other.
To clarify which versions of node-sass are compatible with which Node.js versions, the table below is provided:
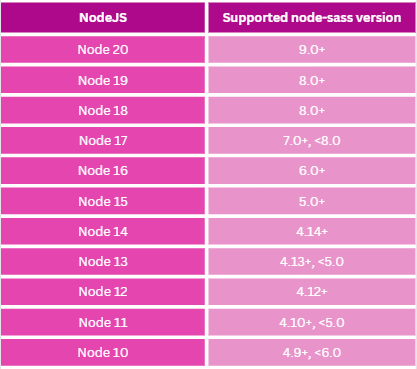
Make sure you use a version of Node.js that works well with the version of node-sass you are using. Check if there are newer versions of node-sass available as it keeps your stylesheets (Sass files) looking sharp and helps you avoid any bugs or issues. Updating node-sass can sometimes bring changes that don’t match your existing styles. To adapt, use a ‘migration strategy,’ a plan to adjust your code, making it work smoothly with the new version of node-sass.
Conclusion
Node-sass makes our websites look great. It takes special styling instructions (Sass) and turns them into something web browsers understand. We’ve covered a lot in this article – from what Sass is, how it works, and its important features, to installing, configuring, and using it in a real-life project.
Node-sass is a useful tool for making your website look good and load fast. But be careful when updating, as it might change things. Make a plan (migration) to adjust your website’s code and keep it working with the latest node-sass version.
If you plan to use node-sass in your projects, make sure you are away from errors and if by chance you get caught, refer to our Sass related error resolution articles:
- Fixing Node Sass Binding Error: Causes and Solutions
- Resolving ‘error /node_modules/node-sass: Command failed’ Error in Node.js
Reference
https://www.npmjs.com/package/node-sass