React Error Boundary is a crucial concept to understand in React development. It provides a robust mechanism for handling errors in a React application, ensuring that an error in one part of the UI doesn’t break the entire application. This article introduces error boundaries, their importance, and how to effectively implement them in your React applications.
What is React Error Boundary?
An error boundary is a special React component that catches JavaScript errors anywhere in their child component tree, logs those errors, and displays a fallback UI instead of the component tree that crashed. It’s like a try/catch block, but for React components.
Error boundaries catch errors during rendering, in lifecycle methods, and in constructors of the whole tree below them. However, they don’t catch errors inside event handlers. React doesn’t need error boundaries to recover from errors in event handlers. Unlike the render method and lifecycle methods, the event handlers don’t happen during rendering.
Why Use Error Boundaries?
Before the introduction of error boundaries in React 16, any JavaScript error in a part of the UI would lead to React unmounting the whole component tree. This wasn’t an ideal user experience. The introduction of error boundaries provided a way to handle such scenarios gracefully.
With error boundaries, you can maintain the application’s stability and integrity by ensuring that the entire UI doesn’t crash if one part encounters an error. You can catch and log errors for further analysis and present a user-friendly error message or a fallback UI to the user.
How to Implement React Error Boundary
Creating an error boundary component
To create an error boundary component, you need to define a new component with either or both of the lifecycle methods getDerivedStateFromError()
or componentDidCatch()
.
The getDerivedStateFromError()
method is used to render a fallback UI after an error is thrown, while the componentDidCatch()
method is used to log error information.
Using the Error Boundary Component
Once you’ve defined your error boundary component, you can use it anywhere in your application like a regular component. It’s usually a good idea to place it near the top of your component tree, because it catches errors in all the components beneath it.
However, you can also use multiple error boundaries in one application. This way, if one component fails, the rest of the application can continue to function normally.
Best Practices for Using React Error Boundary
Placement of error boundaries
While you can place your error boundaries anywhere in your component tree, it’s generally a good practice to place them at the top of your component hierarchy. This ensures that an error in any component doesn’t crash the entire application.
However, you can also use multiple error boundaries to encapsulate different parts of the application. This can be beneficial in large applications where different sections can function independently.
Error reporting
Error boundaries provide a great way to catch and handle errors in React. However, they shouldn’t be used as a way to hide or ignore errors. It’s important to log these errors and fix them.
You can use tools like Sentry, LogRocket, or TrackJS to catch and report errors in production. These tools can provide valuable insights into the error, such as the component stack trace, which can help in debugging.
Limitations of React Error Boundary
While React Error Boundary is a powerful tool for error handling in React, it does have some limitations. It doesn’t catch errors for:
- event handlers
- asynchronous code
- server-side rendering
- errors thrown in the error boundary itself
To catch errors in these cases, you’d need to use traditional JavaScript error handling methods like try/catch.
Conclusion
React Error Boundary is a vital tool for maintaining the stability and user experience of your React applications. By understanding and effectively implementing error boundaries, you can ensure that your application is robust and resilient to JavaScript errors.
Remember, while error boundaries enhance your application’s error handling capabilities, they aren’t a substitute for good coding practices and thorough testing. Always strive to write error-free code and handle errors gracefully when they do occur.
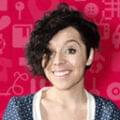
Dianne is SitePoint's newsletter editor. She especiallly loves learning about JavaScript, CSS and frontend technologies.