The period_range() function is used to generate a sequence of periods within a specified range with a fixed frequency.
Whenever we have data in the form of time-indexing, the period_range() will always help us to generate the specific periods within a date range. period_range() makes it very easy to generate the periods in finance where months or quarter time periods are the most common way to analyze the financial data. period_range() is also very useful when someone performs time-based aggregation of data, also in data visualization period_range() plays a very important role in creating a regular sequence of periods.
In this article, we will look at a few ways to generate a period range using Pandas period_range() function. Let’s get started.
Also Read: Create a Pandas DataFrame from Lists (5 Easy Approaches)
Pandas period_range() Method
In Python, the period_range() is a powerful tool the Pandas library provides. A period in Pandas represents a fixed-frequency interval, such as a day, month, or year. The period_range() is particularly useful for working with time-based data that needs to be represented in regular intervals.
Syntax:
pandas.period_range(start=None, end=None, periods=None, freq=None, name=None)
where:
- start: The start date of the period range. It can be a string, period-like, or DateTime object. If not specified, it defaults to None.
- end: The end date of the period range. It can be a string, period-like, or DateTime object. If not specified, it defaults to None.
- periods: The number of periods to generate. If start and end are not provided, periods must be specified. The period range will be generated using equally spaced intervals between the start and end.
- freq: The frequency of the period range. It can be a string or a DateOffset object, specifying the intervals between periods. Common values include ‘D‘ for daily, ‘M‘ for monthly, ‘A‘ for annual, etc.
- name: A name to assign to the generated PeriodIndex.
Implementing Pandas period_range() Method
The period_range() method allows us to create period ranges in various ways which few ways are as follows:
- By specifying start, freq, and periods
- By specifying start, end, and freq
- By specifying start, end, freq, and name
1. By specifying start, freq, and periods
This will generate a sequence of periods from the start with a fixed number of periods and with the specified freq.
Example 1:
import pandas as pd
per = pd.period_range("01/01/22", freq ="M", periods =12)
print(per)
Here first we have imported the Pandas library in pd. Then we have provided the start as 01/01/22, frequency(freq) as Month(M), and periods as 12 into the pd.period_range( ).
Then we printed the pd.period_range( ) function and we saw that it printed twelve different months starting from 01/02/22 with the frequency of one month.
Output:

Example 2:
import pandas as pd
per = pd.period_range("2022",freq="Y", periods=12)
print(per)
Here we provided the start as 2022, freq as Y, and periods as 12 into pd.period_range( ) function . Then we printed the function and we saw that we got twelve different years starting from 2022 with the frequency of one year.
Output:

Example 3:
import pandas as pd
per = pd.period_range("2022",freq="Q", periods=12)
print(per)
Here we provided the start as 2022, freq as Q, and periods as 12 into pd.period_range( ) function. Then we printed the function and we have seen that we got twelve different years starting from 2022Q1 with the frequency of quarter of the year.
Output:

2. By specifying start, end, and freq
This will generate a sequence of periods from the start to the end with the specified freq.
Example:
import pandas as pd
per = pd.period_range(start=pd.Period('2022Q1',freq="Q"),end=pd.Period('2022Q2',
freq="Q"), freq="M")
print(per)
Here we provided the start as pd.Period(‘2022Q1’,freq=”Q”), end as pd.Period(‘2022Q2’, freq=”Q”) and freq as M. Then after printing the pd.period_range( ) function we can see that we got four different months from the first quarter of 2022 to the second quarter of 2022 with the frequency of one month.
Output:

3. By specifying start, end, freq, and name
This will generate a sequence of periods from the start to the end with the specified freq and name.
Example:
import pandas as pd
per = pd.period_range(start="01/01/2022", end ="31/03/2022",freq ="W", name="weekwisefrequency")
print(per)
Here we have provided the start as 01/01/2022, end as 31/03/2022, freq as W, and name as weekwisefrequency. After printing the pd.period_range( ) function we got the dates from start to end with the frequency weekly wise and the name weekwisefrequency.
Output:
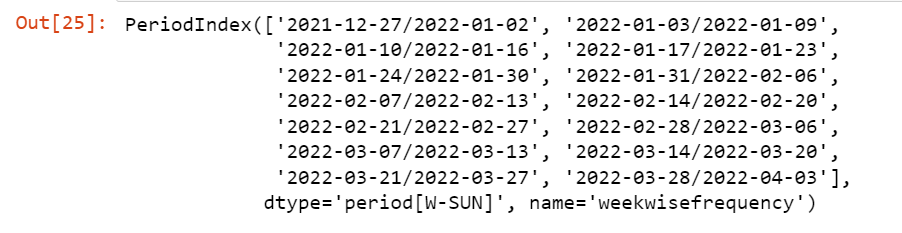
Summary
The period_range() function in Python’s Pandas library is a valuable tool for working with time-based data in regular intervals. It simplifies tasks like time-based aggregation, time series analysis, data filtering, and plotting, making it easier to handle and analyze time-indexed data. We have discussed three ways to generate a period range. After reading this tutorial we hope you can easily generate a period range using Pandas in Python.