Using Faker to Generate Filler Data for Automated Testing


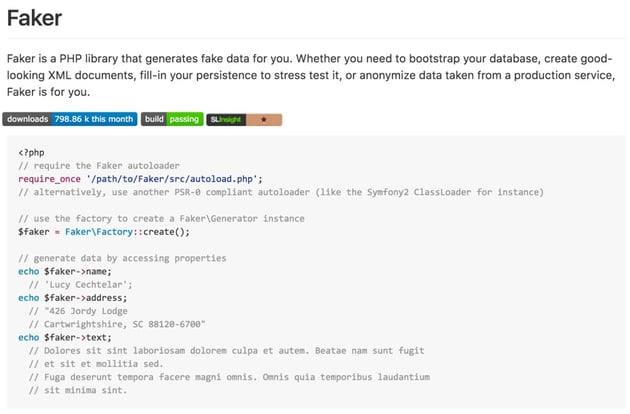

The development of some websites and applications requires the use of different types of data to simulate how something would work in real life. During the testing and development phase of a project, we often use fake data to fill up our database, populate UI elements, and so on.
Writing the code to generate fake data for your project yourself can be quite tiresome. In this tutorial, you will learn how to use the tried and tested Faker library in PHP to generate fake data.
Getting Started
Before proceeding further, I would like to clarify a few things.
The original fake library was fzaninotto/Faker. However, it was archived by the owner on 11 December 2020. A fork of the library called FakerPHP/Faker is now taking the development work forward. If you are trying to decide which of these you should be using in your project, pick FakerPHP.
The Faker from FakerPHP requires your PHP version to be greater than to equal to 7.4. This is in contrast to PHP >= 5.3.3 supported by the original library.
From now on, all references to Faker in this tutorial will be meant for the newer forked version.
You can install faker using composer by running the following command:
1 |
composer require fakerphp/faker |
This will create a composer.json file for you and install all the required packages inside the vendor directory.
Once it's installed, you can start using the library in your project by adding these two lines to your code:
1 |
<?php
|
2 |
|
3 |
require 'vendor/autoload.php'; |
4 |
$faker = Faker\Factory::create(); |
The first line includes the faker library in our project.
In the second line, we initialize a faker generator with a call to the static create()
method of the Factory
class from the Faker
namespace. This gives us a Generator
instance that we can use to generate all kinds of fake data.
Generating Fake Names, Addresses, and Phone Numbers
The Generator
we instantiated in the previous section requires a Provider
object to generate random data. A call to the create()
method above created a Generator
instance for us that was bundled with the default providers.
The default provider generates US-based names, addresses, phone numbers, company details, etc. These calls add the provider under the hood:
1 |
$faker = new Faker\Generator(); |
2 |
$faker->addProvider(new Faker\Provider\en_US\Person($faker)); |
3 |
$faker->addProvider(new Faker\Provider\en_US\Address($faker)); |
4 |
$faker->addProvider(new Faker\Provider\en_US\PhoneNumber($faker)); |
5 |
$faker->addProvider(new Faker\Provider\en_US\Company($faker)); |
6 |
$faker->addProvider(new Faker\Provider\Lorem($faker)); |
7 |
$faker->addProvider(new Faker\Provider\Internet($faker)); |
You can generate fake names in faker by simply making a call to the name()
method or accessing the name
property. Each call will output a different random name. Here is an example:
1 |
<?php
|
2 |
|
3 |
require 'vendor/autoload.php'; |
4 |
$faker = Faker\Factory::create(); |
5 |
|
6 |
$fake_names = []; |
7 |
for($i = 0; $i < 10; $i++) { |
8 |
$fake_names[] = $faker->name; |
9 |
}
|
10 |
|
11 |
/*
|
12 |
Array
|
13 |
(
|
14 |
[0] => Gabe Mann Jr.
|
15 |
[1] => Lazaro Leuschke
|
16 |
[2] => Angie Welch Sr.
|
17 |
[3] => Prof. Kirk Krajcik III
|
18 |
[4] => Sadye Mosciski
|
19 |
[5] => Danyka Braun
|
20 |
[6] => Jacinthe Dickinson
|
21 |
[7] => Clifton Beahan
|
22 |
[8] => Dr. Jan Casper MD
|
23 |
[9] => Adelia Schimmel
|
24 |
)
|
25 |
*/
|
26 |
print_r($fake_names); |
27 |
|
28 |
?>
|
If you aren't interested in full names, you can also ask for just the first name or last name.
1 |
<?php
|
2 |
|
3 |
require 'vendor/autoload.php'; |
4 |
$faker = Faker\Factory::create(); |
5 |
|
6 |
$first_names = []; |
7 |
for($i = 0; $i < 10; $i++) { |
8 |
$first_names[] = $faker->firstName; |
9 |
}
|
10 |
|
11 |
// Outputs: Dylan, Ariane, Doris, Reilly, Jamar, Merl, Maverick, Frederik, Lucius, Madyson
|
12 |
echo implode(', ', $first_names); |
13 |
|
14 |
?>
|
Let's say you only want male or female names. In this case, you can simply make a call to the name()
method and pass the gender as either male or female. Alternatively, you could make a call to dedicated methods firstNameMale()
and firstNameFemale()
. Here is an example:
1 |
<?php
|
2 |
|
3 |
require 'vendor/autoload.php'; |
4 |
$faker = Faker\Factory::create(); |
5 |
|
6 |
$first_names = []; |
7 |
for($i = 0; $i < 10; $i++) { |
8 |
if($i < 5) { |
9 |
$first_names[] = $faker->firstName('female'); |
10 |
} else { |
11 |
$first_names[] = $faker->firstNameFemale(); |
12 |
}
|
13 |
}
|
14 |
|
15 |
// Outputs: Janae, Lavada, Kelly, Isabella, Lucy, Roxane, Opal, Abagail, Karlee, Laney
|
16 |
echo implode(', ', $first_names); |
17 |
|
18 |
?>
|
Similarly, you can generate fake US addresses by calling the address()
method or accessing the address
property.
1 |
<?php
|
2 |
|
3 |
require 'vendor/autoload.php'; |
4 |
$faker = Faker\Factory::create(); |
5 |
|
6 |
/*
|
7 |
93432 Rodger Gateway Suite 092
|
8 |
Gorczanyburgh, CO 14433-1354
|
9 |
|
10 |
4755 Weimann Mission
|
11 |
Dalemouth, MO 62775
|
12 |
|
13 |
6108 Thalia Vista
|
14 |
New Mabelleside, MA 58031-4497
|
15 |
*/
|
16 |
for($i = 0; $i < 3; $i++) { |
17 |
echo $faker->address()."\n\n"; |
18 |
}
|
19 |
|
20 |
?>
|
If you want a random city name, state name, street name, or postal code, you can also call the respective methods.
1 |
<?php
|
2 |
|
3 |
require 'vendor/autoload.php'; |
4 |
$faker = Faker\Factory::create(); |
5 |
|
6 |
/*
|
7 |
Carmenshire, Maine, Alan Parks, 47463
|
8 |
Kochmouth, Colorado, Brooke Courts, 70222
|
9 |
Collierview, Illinois, Effertz Fords, 59033-1508
|
10 |
*/
|
11 |
for($i = 0; $i < 3; $i++) { |
12 |
echo $faker->city.", ".$faker->state.", ".$faker->streetname.", ".$faker->postcode."\n"; |
13 |
}
|
14 |
|
15 |
?>
|
You can also generate different types of random phone numbers by calling phoneNumber()
, tollFreePhoneNumber()
, or phoneNumberWithExtension()
.
1 |
<?php
|
2 |
|
3 |
require 'vendor/autoload.php'; |
4 |
$faker = Faker\Factory::create(); |
5 |
|
6 |
/*
|
7 |
Phone Number: +1-724-494-3101
|
8 |
Phone Number (Extension): (475) 499-3999 x9969
|
9 |
Phone Number (Toll Free): 855.618.0155
|
10 |
|
11 |
Phone Number: 1-660-934-8668
|
12 |
Phone Number (Extension): 351-533-3301 x1602
|
13 |
Phone Number (Toll Free): 844-530-4671
|
14 |
*/
|
15 |
for($i = 0; $i < 2; $i++) { |
16 |
echo "Phone Number: ".$faker->phoneNumber()."\n"; |
17 |
echo "Phone Number (Extension): ".$faker->phoneNumberWithExtension()."\n"; |
18 |
echo "Phone Number (Toll Free): ".$faker->tollFreePhoneNumber()."\n\n"; |
19 |
}
|
20 |
|
21 |
?>
|
Generating Locale-Specific Fake Details
Let's say you are developing an app that targets a specific region. In this case, you might want to use data that reflects your target market. For example, an application meant for Nigerian people will benefit from having Nigerian names. Similarly, an app for Indian users might want to use Indian addresses.
The trick to generate locale-specific data is to pass the locale to the create()
method. Here is an example:
1 |
<?php
|
2 |
|
3 |
require 'vendor/autoload.php'; |
4 |
|
5 |
$nigerian_faker = Faker\Factory::create('en_NG'); |
6 |
$indian_faker = Faker\Factory::create('en_IN'); |
7 |
$french_faker = Faker\Factory::create('fr_FR'); |
8 |
|
9 |
/*
|
10 |
Nigerian Name: Chizoba Chiamaka Oluwaseyi
|
11 |
Nigerian Address: 30 Ayomide Street 66 790 OmolaraVille
|
12 |
*/
|
13 |
echo "Nigerian Name: ".$nigerian_faker->name."\n"; |
14 |
echo "Nigerian Address: ".$nigerian_faker->address."\n\n"; |
15 |
|
16 |
/*
|
17 |
Indian Name: Yadunandan Goda
|
18 |
Indian Address: 74, Ragini Society, Ehsaan Nagar Trichy - 583865
|
19 |
*/
|
20 |
echo "Indian Name: ".$indian_faker->name."\n"; |
21 |
echo "Indian Address: ".$indian_faker->address."\n\n"; |
22 |
|
23 |
/*
|
24 |
French Name: Pierre Neveu
|
25 |
French Address: 32, chemin Agathe Bouvier
|
26 |
05574 NoelVille
|
27 |
*/
|
28 |
echo "French Name: ".$french_faker->name."\n"; |
29 |
echo "French Address: ".$french_faker->address."\n"; |
30 |
|
31 |
?>
|
Generating Random Numbers and Strings
You can also use Faker to generate other types of random data. This includes random numbers, strings, digits, or letters. The library also provides methods for picking one or more random elements from an array or shuffling the elements in a string or array.
1 |
<?php
|
2 |
|
3 |
require 'vendor/autoload.php'; |
4 |
|
5 |
$faker = Faker\Factory::create(); |
6 |
|
7 |
$r_nums = []; |
8 |
$r_letters = []; |
9 |
$r_nums_four_dgt = []; |
10 |
$r_nums_in_range = []; |
11 |
|
12 |
for($i = 0; $i < 5; $i++) { |
13 |
$r_nums[] = $faker->randomNumber(4, false); |
14 |
$r_letters[] = $faker->randomLetter(); |
15 |
$r_nums_four_dgt[] = $faker->randomNumber(4, true); |
16 |
$r_nums_in_range[] = $faker->numberBetween(100, 500); |
17 |
}
|
18 |
|
19 |
// Output: 6386, 215, 1911, 965, 1757
|
20 |
echo implode(", ", $r_nums)."\n"; |
21 |
|
22 |
// Output: 7910, 2427, 3725, 6776, 2771
|
23 |
echo implode(", ", $r_nums_four_dgt)."\n"; |
24 |
|
25 |
// Output: 296, 327, 337, 198, 404
|
26 |
echo implode(", ", $r_nums_in_range)."\n"; |
27 |
|
28 |
// Output: y, w, e, f, g
|
29 |
echo implode(", ", $r_letters); |
30 |
|
31 |
// Output: f, y
|
32 |
echo implode(", ", $faker->randomElements($r_letters, 2)); |
33 |
|
34 |
?>
|
The randomNumber()
method generates random numbers with a specified number of digits by default. You can pass false
as the second parameter to generate random numbers that contain between zero and the specified number of digits.
Generating Random Dates
The library also has quite a few methods for generating random dates in a variety of formats.
You can use the unixTime()
method to get a Unix timestamp value between 0 and the current or specified time. It is also possible to generate a random DateTime
object between 1 January 1970 and the given maximum value using the dateTime()
method. Again, the default maximum is the current date and time.
If you want to generate DateTime
objects that go back further into the past, you can consider using the dateTimeAD()
method, which gives back dates between 1 January 0001 and the given maximum value.
You can use the date()
and time()
methods to generate random dates and times with a specific format.
Let's say you need to get a DateTime
object that falls between two specified dates. You can do so by using the dateTimeBetween()
method.
1 |
<?php
|
2 |
|
3 |
require 'vendor/autoload.php'; |
4 |
|
5 |
$faker = Faker\Factory::create(); |
6 |
|
7 |
/*
|
8 |
object(DateTime)#25 (3) {
|
9 |
["date"]=>
|
10 |
string(26) "1973-04-09 03:51:25.000000"
|
11 |
["timezone_type"]=>
|
12 |
int(3)
|
13 |
["timezone"]=>
|
14 |
string(13) "Europe/Berlin"
|
15 |
}
|
16 |
*/
|
17 |
var_dump($faker->dateTime())."\n"; |
18 |
|
19 |
/*
|
20 |
object(DateTime)#25 (3) {
|
21 |
["date"]=>
|
22 |
string(26) "1211-08-03 21:40:42.000000"
|
23 |
["timezone_type"]=>
|
24 |
int(3)
|
25 |
["timezone"]=>
|
26 |
string(13) "Europe/Berlin"
|
27 |
}
|
28 |
*/
|
29 |
var_dump($faker->dateTimeAD())."\n"; |
30 |
|
31 |
// Outputs: 2007-04-30
|
32 |
echo $faker->date()."\n"; |
33 |
|
34 |
// Outputs: 23 December, 1985
|
35 |
echo $faker->date('j F, Y')."\n"; |
36 |
|
37 |
// Outputs: 06:56:49
|
38 |
echo $faker->time()."\n"; |
39 |
|
40 |
/*
|
41 |
object(DateTime)#25 (3) {
|
42 |
["date"]=>
|
43 |
string(26) "2023-07-25 10:47:32.000000"
|
44 |
["timezone_type"]=>
|
45 |
int(3)
|
46 |
["timezone"]=>
|
47 |
string(13) "Europe/Berlin"
|
48 |
}
|
49 |
*/
|
50 |
var_dump($faker->dateTimeBetween('-1 month', '+1 month'))."\n"; |
51 |
|
52 |
?>
|
Generating Markup With Fake Data
Now that we know how to generate different types of values using Faker, we can combine their result to create XML or HTML documents, fill a database with values, and so on. For example, consider the code below, which generates random profiles for different people.
1 |
<?php
|
2 |
|
3 |
require 'vendor/autoload.php'; |
4 |
|
5 |
$faker = Faker\Factory::create(); |
6 |
|
7 |
/*
|
8 |
<div class="profile">
|
9 |
<p>Name: Melissa Smitham</p>
|
10 |
<p>Date of Birth: April 9, 2022</p>
|
11 |
<p>Height: 194 cms.</p>
|
12 |
<p>Residence: North Callieton, New York</p>
|
13 |
</div>
|
14 |
|
15 |
<div class="profile">
|
16 |
<p>Name: Brennon Schultz III</p>
|
17 |
<p>Date of Birth: February 4, 1995</p>
|
18 |
<p>Height: 160 cms.</p>
|
19 |
<p>Residence: Shieldston, Nebraska</p>
|
20 |
</div>
|
21 |
|
22 |
<div class="profile">
|
23 |
<p>Name: Domingo Halvorson</p>
|
24 |
<p>Date of Birth: November 20, 1970</p>
|
25 |
<p>Height: 162 cms.</p>
|
26 |
<p>Residence: West Damonton, New York</p>
|
27 |
</div>
|
28 |
*/
|
29 |
for($i = 0; $i < 3; $i++) { |
30 |
$name = $faker->name; |
31 |
$dob = $faker->dateTime()->format('F j, Y'); |
32 |
$height = $faker->numberBetween(160, 200); |
33 |
$city_state = $faker->city.", ".$faker->state; |
34 |
|
35 |
echo <<<PROFILE |
36 |
<div class="profile"> |
37 |
<p>Name: $name</p> |
38 |
<p>Date of Birth: $dob</p> |
39 |
<p>Height: $height cms.</p> |
40 |
<p>Residence: $city_state</p> |
41 |
</div> |
42 |
|
43 |
|
44 |
PROFILE; |
45 |
}
|
46 |
|
47 |
?>
|
Once the random values have been generated, you can do anything with them. In this case, we output them inside a div
element to create profiles of three different people.
Final Thoughts
We have only scratched the surface when it comes to the capabilities of the Faker library. You can use the library to generate all kinds of fake data, such as images, user agents, paragraphs, colors, email address, domains, and so on. For some types of data, such as a random color value, you can get the final result in different formats such as Hex, RGB, RGBA, HSL, or a CSS-friendly color name.
The library also offers the flexibility of creating custom providers that extend the Base
class to generate the type of data we want. Faker is definitely worth checking out if you are looking for a library that generates random data.