Object Oriented Programming is a programming paradigm that is centered around objects rather than functions. Before OOP we had procedural programming where the program is divided into several functions. We had data stored in different variables and functions and worked on those data to produce results. There was too much interdependence in this system. As a solution to this problem, OOP was developed. In OOP there are three important concepts: 1. Abstraction, 2. Inheritance and 3. Polymorphism. Today, in this article we will learn about the second concept: Inheritance.
Concept of OOP
In OOP we combine a group of related variables and functions into an object. We refer to these variables as properties of the object and refer to the functions as methods, this is known as encapsulation. We have mentioned the 3 main concepts in OOP, let us look at them once again.
- Abstraction: Make a simpler interface. To understand the notion of this concept thinks of your program as a complex electronic device, say a microwave oven. As a user you can only interact with the control panel of the microwave, you do not need to understand the inner workings of the machine in order to successfully get results, this is what we achieve with the help of abstraction. We only make the absolutely necessary parameters available to the end user and nothing else. This also reduces any effect of change in any of the parameters
- Inheritance: This allows us to eliminate redundant code.
- Polymorphism: Poly means many, and morph means form, so as a whole it literally translates to many forms. This allows us to eliminate a lot of if-else and switch-case statements and makes for clean code.
What is Inheritance?
To understand Inheritance first we need to understand the concept of a Class. A Class is basically a template for creating any objects. Inside each class, there is a compulsory constructor method that is responsible for creating properties whenever a new object is created for that class. Let us understand how to create a Class with the help of code:
Say we want to create a Cars object and a Truck object, now say each of these has the following three properties: driverName, dateOfManufacture and topSpeed. Now we can clearly see that we would have to write the three properties for each of the objects we create, this is a bad practice. A more efficient practice is to create a common Class and create these objects from that class, let’s look at the code then.
Let us create a Vehicle Class, containing the above three properties, as well as a function for calculating the topSpeed in Miles.
console.log("this is inheritance!")
class Vehicle{
constructor(driverName, dateOfManufacture, topSpeed) {
this,driverName = driverName;
this.dateOfManufacture = dateOfManufacture;
this.topSpeed = topSpeed;
}
topSpeedInMiles() {
this.topSpeed = this.topSpeed * 0.6;
}
}
Now let us create the car and the truck objects and call the topSpeedInMiles() function and look at the results again:
const car = new Vehicle("Anubhav", "23-8-90", 100);
console.log(car);
car.topSpeedInMiles();
console.log(car);
// const truck = new Vehicle("Rohit", "10-4-16", 200);
// console.log(truck);
// truck.topSpeedInMiles();
// console.log(truck);
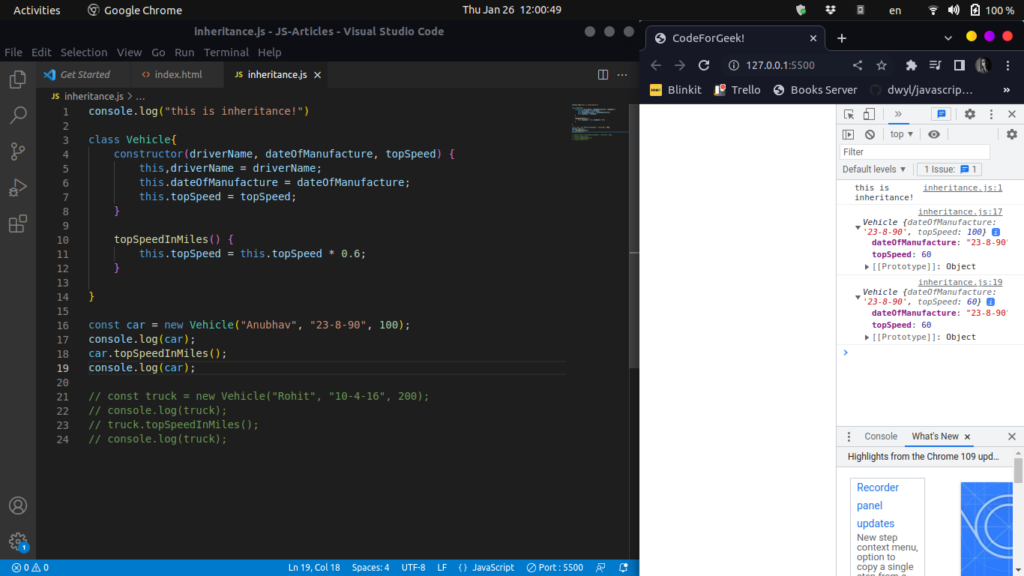
The important thing to notice here is that the three properties and a method are written only once in the parent Class and all objects created from that class (car and truck) have all of these properties and the method available from the start, they inherited these properties and methods from the parent Class. This is the concept of inheritance. On top of this, we can add any new method or property to these objects, let us see how to do that now.
We can create a new class for both cars and trucks, for now, let us just concentrate on one of these: cars. We would like to have all of the properties of the Vehicle class as well have some new properties and methods. Let us add a new method to the Vehicle Class called startVehicle which will console out: “the cars has started”. Now, let us also create a method in the child class Car called startCar which will console log something else.
Now if we create a new object using the Car Class, we can not only call the startCar method but also the startVehicle method as well, this is an inherited method.
class Vehicle{
constructor(driverName, dateOfManufacture, topSpeed) {
this,driverName = driverName;
this.dateOfManufacture = dateOfManufacture;
this.topSpeed = topSpeed;
}
startVehicle() {
console.log("Vehicle has started");
}
topSpeedInMiles() {
this.topSpeed = this.topSpeed * 0.6;
}
}
class Car extends Vehicle{
startCar() {
console.log("BRRRRRRR!")
}
}
const i10 = new Car();
i10.startCar();
i10.startVehicle();
Conclusion
We really hope that you guys understood the concept of inheritance in JavaScript. All of the code is written using ES6 which is a must if you are opting for a career in web dev now. Alright, if you are looking for more such tutorials you know where to find us, all the best!
Reference
https://developer.mozilla.org/en-US/docs/Learn/JavaScript/Objects/Classes_in_JavaScript