Today we’re doing something more practical and interesting. We’re building a search engine with an Elasticsearch module in our Nodejs application!
Elasticsearch is so versatile if you had to ask 10 different developers ‘What is Elasticsearch?’, each one of them would give you a different answer. And, none of them are wrong! It has grown as a solution to many programming problems because of its proliferating popularity and versatility.
So, before we get started with building a search engine with Elasticsearch for our Nodejs project, let us get into some details about Elasticsearch.
What is Elasticsearch?
Well, this is a question that has got 10 or even 20 different answers to it. But if I were to tell you an all-encompassing definition as a beginner, I could tell you it is an open-source, distributable search engine and analytics tool.
It is built on top of Apache Lucene and stores data in JSON format in a document-based structure. More like NoSQL databases like MongoDB, Cassandra, and Redis.
Elasticsearch lets you store and look up a gigantic amount of data in almost real-time. With a REST API available for its storing and searching operations, Elasticsearch is highly scalable.
It features a distributed backend that allows distributing tasks, includes search, indexing, as well as for analytics across a cluster of nodes.
Now that we have a somewhat clear picture of what Elasticsearch is, let us jump to building our search engine with Elasticsearch in Nodejs.
Getting Started with Building a Search Engine with Elasticsearch
Before we get started with creating a search engine with Elasticsearch, I’d like to lay down some important prerequisites this section assumes you have fulfilled.
- You have Nodejs installed on your machine, if not please visit the official download page.
- You have installed Elasticsearch on your machine. If not, please visit the official download page.
Once you have installed Elasticsearch, it is better to make sure it was properly installed by sending a request to the Elasticsearch server like this:
curl http://127.0.0.1:9200
You can use Postman to check if it is working. If you see status 200 OK, then it is clearly working.
Now, let us create a new Nodejs application at this point. Also, create your entry point .js file; I am calling mine app.js.
Now let’s install the packages that we need to build our search engine with Elasticsearch. We are using the Node Elasticsearch client module known as elasticsearch. Hence, you will also have to install the body-parser package along.
Most code snippets in this section are taken from LiveCodeStream.
npm install express elasticsearch body-parser
Let us set up our app.js as usual:
const express = require(‘express’) const bodyParser = require(‘body-parser’) const elasticsearch = require(‘elasticsearch’) const app = express() app.use(bodyParser.json()) app.listen(8000, () => { console.log(‘App running on port 8000.’) })
Let us now move on to the next steps of creating and setting up an Elasticsearch client.
const esClient = elasticsearch.Client({ host: "http://127.0.0.1:9200", })
Let us now proceed to create a /POST route to ‘/products’. It will accept POST requests to help Elasticsearch index new products into an index called ‘products’.
Index in Elasticsearch, refers to a collection of documents. The concept here is quite different from what we learn in databases like MySQL.
For this, we can make use of the index method in the elasticsearch module, like this:
app.post("/products", (req, res) => { esClient.index({ index: 'products', body: { "id": req.body.id, "name": req.body.name, "price": req.body.price, "description": req.body.description, } }) .then(response => { return res.json({"message": "Indexing successful"}) }) .catch(err => { return res.status(500).json({"message": "Error"}) }) })
Now our ‘products’ index doesn’t exist when we first start our server. However, the first request to this route will remind Elasticsearch to create the new index.
Let us now send a new request and test this route using Postman. if you see the response message as ‘Indexing successful’, which is we set up in our code up there, it means your application is working just fine!
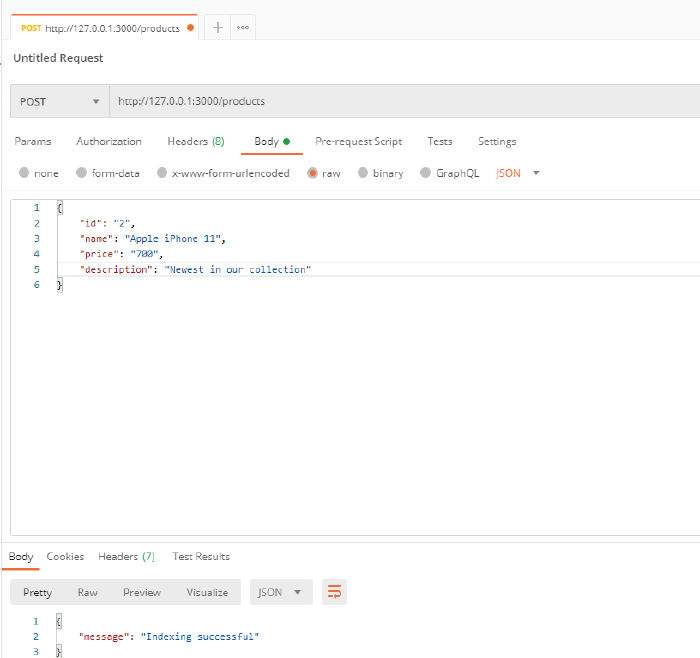
We have also indexed a few more new products with words like Apple and iPhone to test how Elasticsearch responds to full-text queries. It is recommended that you also try and index some products with similar names to make sure everything is working.
Moving forward to our next step in building a search engine with Elasticsearch, let us now create a GET /products route. As you must have already guessed, this route will be used for handling the GET requests with text queries for a product a user might search for.
Our application server should be able to respond with a list of products that match the user’s search. For this, we are using this text query to search the name fields of the products indexed in Elasticsearch.
app.get("/products", (req, res) => { const searchText = req.query.text esClient.search({ index: "products", body: { query: { match: {"name": searchText.trim()} } } }) .then(response => { return res.json(response) }) .catch(err => { return res.status(500).json({"message": "Error"}) }) })
You can now test this route to see if it is working. Let us use a search phrase called “blue iphone backcover”.
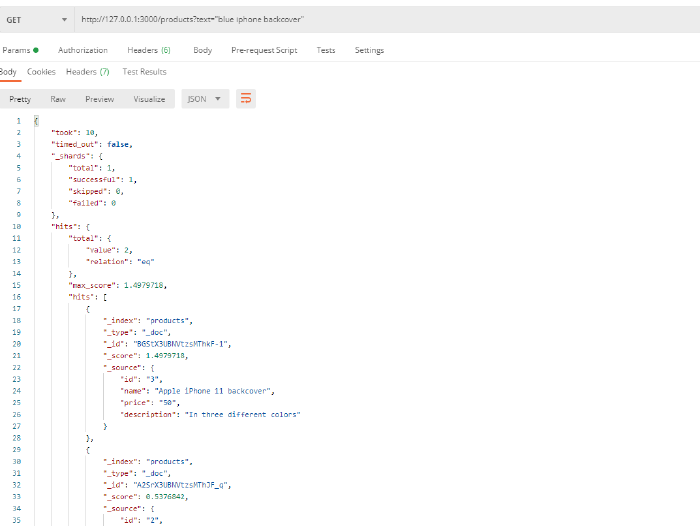
As you can see, our server responds with two products, the first one is the “Apple iPhone 11 backcover” and the other is the “Apple iPhone 11”.
Although the second result is not quite what the user searched for, Elasticsearch still considered it as a hit because it indeed matched with one other word in the search phrase called ‘iphone’. And you can notice it is listed after the result which is more relevant to what we searched.
This way, you can go about building a search engine with Elasticsearch for your beautiful and awesome Nodejs applications.
If you want to learn how to integrate MongoDB and Elasticsearch together in your Nodejs application, read this tutorial and create a search engine with Elasticsearch.
Conclusion
This tutorial is a walkthrough on building a search engine with Elasticsearch in Nodejs. Read this easy step-by-step guide to build search engines of your own for your stunning NodeJS applications.