It has been a year since I’ve been exploring the gRPC framework. Most of the time, I’ve written web services in gRPC and glued them together with their implementations. Most of the time without having to explore it why and how it came into the light? I find people relating the gRPC framework to the “Microservices” world. If you dig on the Internet, you can find written materials like “Microservice at Scale with gRPC framework” or “Building scalable Microservices with gRPC”.
Why Read this blog?
If you are trying to answer questions like:
- what “gRPC” is and why does it fundamentally exist,
- How request/response format matters to Web service performance? you should continue to read this blog.
I will also walk through a brief history of the distributed computation and will come to the definition of the remote procedure call and finally we will switch why “gRPC” exists.
What is gRPC?
Let me be a lazy and refer you to the wiki’s definition of what a gRPC is?
gRPC (gRPCRemote Procedure Calls[1]) is an open sourceremote procedure call (RPC) system initially developed at Google in 2015[2]. It uses HTTP/2 for transport, Protocol Buffers as the interface description language, and provides features such as authentication, bidirectional streaming and flow control, blocking or non-blocking bindings, and cancellation and timeouts.
Before we describe gRPC in detail, let us understand what a Remote Procedure Call is and a little bit of history of distributed programming.
A Flashback of Remote Procedure Call
It was a protocol for distributed programming and computer networks developed by Bruce J. Nelson. It coined the idea of invoking methods or programs residing on a remote machine. Although the theoretical terms related to distributed programming have been there from the 1960s one of the practical implementations of RPC came in light in the 1980s with a paper named Implementing Remote Procedure Call. When a procedure call is invoked in a normal world (Same machine scenario), the transfer of control and the associated data transferred to the calling method and a return is expected with some computation. In RPC, the same process is extended to the network. With the popularity of object-oriented programming in the 1990s, there were multiple implementations of the same. Having said that, let us quickly recap how some of the famous implementations of the RPC worked. You eagerly waiting to know more about the gRPC, you can simply jump to Protobuf section.
Distributed Computing Environment/Remote Procedure Call (DCE/RPC)
The earliest standard example I could found for RPC implementation is DCE/RPC. You can further dig-in the link for implementation details.
DCE/RPC has the familiar client/server architecture in which a client invokes a procedure that executes on the server. Arguments can be passed from the client to the server and return values can be passed from the server to the client. The framework is platform- and language-neutral in principle, although strongly tilted toward C in practice. DCE/RPC includes utilities for generating client and server artifacts (stubs and skeletons, respectively). DCE/RPC also provides software libraries that hide the transport details.
It uses interface definition language as an agreement for request-response patterns between client and server. for e.g.
/* echo.idl */
[uuid(2d6ead46-05e3-11ca-7dd1-426909beabcd), version(1.0)]
interface echo {
const long int ECHO_SIZE = 512;
void echo(
[in] handle_t h,
[in, string] idl_char from_client[ ],
[out, string] idl_char from_server[ECHO_SIZE]
);
}
As you can notice in the above example, the interface is defined in a “C” like syntax in which the remote procedure “echo” returns nothing but accepts three parameters. First two of these parameters are the input to the procedure while the “out” is the output returned by the server. The payload type of the DCE/RPC protocol is binary and it can run on the top of TCP, UDP, SMB protocols. The protocol used for many remote procedure call-based applications, including many applications from Microsoft. MS-RPCE being one of the RPCs based on the same.
XML-RPC
In the late 1990s, a person named Dave Winer innovated a lightweight language neutral XML based RPC which requires Marshalling and UnMarshalling at the client and server-side in order to convert the object from and to XML as a native object. This protocol supports request/response patterns for distributed programming. As a wire protocol, it uses HTTP. Therefore any system which uses XML-RPC requires an HTTP library to generate, parse, transform the request and respond. For example, look at the below xml which works as a service contract for fib method which accepts a 4 byte Int. The value of the argument “11” is passed as text.

Java RMI
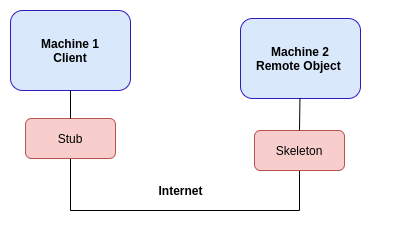
Java released it’s implementation of RPC named Java RMI (Remote Method Invocation). It allowed distributed programming in Java. The simplest diagram for this I could find on the Internet is on JavaPoint. Here we want to call a method on Remote Object residing on Machine 2 from a client residing on Machine 1.
There are two objects here which brokering the request between client and remote object called stub and skeleton.
Here is what stub does:
- Acts as a gateway for the client side.
- Client invokes the remote method through stub
- Initiates a connection with remote, marshalls the request and transmit it to remote JVM and waits for the result.
- It reads (unmarshals) the return value or exception, and
- It finally, returns the value to the caller.
Skeleton
- It reads the parameter for the remote method
- It invokes the method on the actual remote object, and
- It writes and transmits (marshals) the result to the caller.
In the Java 2 SDK, an stub protocol was introduced that eliminates the need for skeletons.
Java RMI wire Protocol
The RMI protocol makes use of two other protocols for its on-the-wire format: Java Object Serialization and HTTP. The Object Serialization protocol is used to marshal call and return data. The HTTP protocol is used to “POST” a remote method invocation and obtain return data
- The wire format is binary which are serialised Java objects.
- The Interface definition language (IDL) is java interfaces.
Apart from these, there were numerous RPC standards and implementation were there but to keep the discussion brief, we will be using the aforementioned RPC implementation in order to understand the gRPC Framework.
gRPC Framework:
Now let us revisit the definition of gRPC in first part of the blog. Because we are now familiar with terms like IDL and Wire format of a RPC protocol, you will be able to relate.
Protocol Buffers:
Protocol buffers are the Interface Definition Language of gRPC.
- Protocol buffers are a flexible, efficient, automated mechanism for serialising structured data – think XML, but smaller, faster, and simpler.
- They are written with a .proto extension. e.g.
syntax = "proto3"; package awake.packet_analysis; message Person { string name = 1; int32 id = 2; string email = 3; }
Protobuf supports flexible type system to support type system available in most of the languages. You can see basic types in the above example such as string and int32 which is equivalent to String and Int in Java. We will go through Protobuf writing specification and encoding in detail as part of the another blog and will see how we generate and use access classes using the Protobuf. For now, let us see how gRPC uses Protobuf which has several advantages over other formats.
Protobufs:
- are simpler
- are 3 to 10 times smaller *
- are 20 to 100 times faster **
- are less ambiguous
- generate data access classes that are easier to use programmatically
Having adopted the Protobuf as wire format for gRPC framework, it adds a lot to performance of the application as well as encourages a Ubiquitous language for Microservices to communicate to each other.
HTTP2
The gRPC framework communicates over HTTP2 connection. The Http2 protocol has improved over its previous version. Here are a few things to count as benefits in layman’s term which I found on this Quora thread.
Constant Connection : HTTP/2 delivers constant connection between client (web/mobile browser) and server that decreases page load time plus it reduce the amount of data being transferred.
Binary Language : It transfer of data in binary language rather then textual format, so computer don’t need to waste time to translate text data into binary format.
Multiplexing: HTTP/2 can send & receive multiple message/data at same time, additionally it also gives features.
- Prioritization : Priority based data transmission, important data will transfer first.
- Compression : It compress the size of data into smaller pieces.
- Server Push : Server makes a pre-guess about the next request & send data.
If you would like to go over little bit of history of how HTTP2 came into picture, you can visit Google developers page.
How gRPC is different?
- Ability to break free from the call-and-response architecture. gRPC is built on HTTP/2, which supports traditional Request/Response model and bidirectional streams.
- Switch from JSON to protocol buffers.
- Multiplexing. (See details here)
- Duplex Streaming. (See how)
- Because of binary data format, it gets much lighter.
- Polyglot (Option to generate access classes in multiple languages.
Having gone through the basics of what a RPC framework is and what gRPC is made of, as part of the next blog in this series, we will create a very basic web service using gRPC framework to understand the concepts practically. Hope you enjoyed reading. Stay tuned!!
References:
