It's common to implement table view which contain cells with different length of text, as the content are retrieved from web APIs.
When implementing a dynamic height tableview cell, one common issue we get is that the text either got clipped or two elements get overlapped :
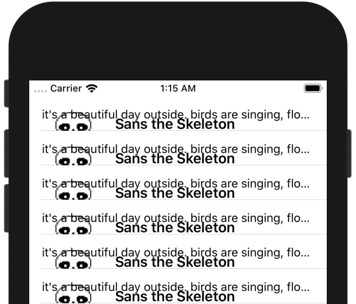
A quick search on google on 'how to make a dynamic height table view cell' will tell you to put in this two line :
tableView.rowHeight = UITableView.automaticDimension
tableView.estimatedRowHeight = 80
Putting these two lines seems easy, but somehow the cell refuse to expand to the right height even when the label exceed one line, and before you know it you have already spent two hours wrangling with the constraints inside the cell and still not working.
The key to making this work is actually setting enough constraints for the elements inside the cell so that Auto layout knows how to calculate the height of the cell.
To let Auto Layout know how to calculate the height of the cell, we need to create sufficient constraints from top of the cell's content view to the bottom.
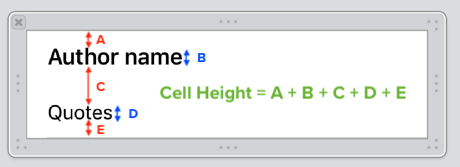
For the cell above with two labels, we need to define the vertical spacing constraint from author name label to the cell content view top (A), the vertical spacing between author name label and quote label (C) and bottom constraint from quote label to the cell content view bottom (E).
A :
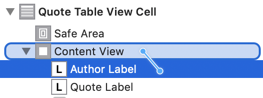

C :
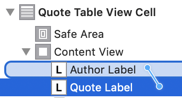

E :
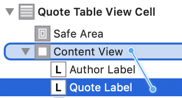

As for the height of author name label (B) and height of quote label (D), we do not need to set a height constraint for them as UILabel has intrinsic content size. UILabel has intrinsic content size, this means that its width and height can be derived from the text string of the label and the font type and font size used.
As long as Auto Layout is able to calculate the height of the cell from top to bottom, it will show dynamic height cell nicely.
The overlapping example at the start of this article happens because I didn't define the vertical spacing constraint between author name label and quote label (C) :
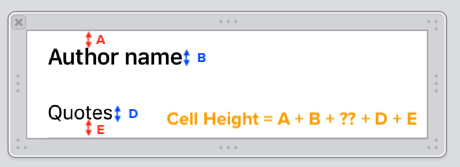
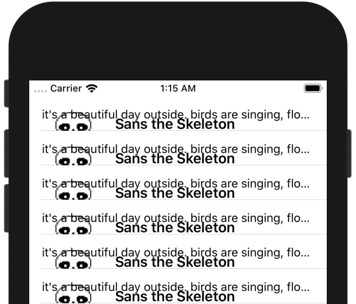
This makes Auto Layout unable to calculate the height of the cell.
When Auto Layout is unable to calculate the height of the cell due to insufficient constraint, it will use the default row height or the row height you have defined in Interface Builder, or the height derived from heightForRowAt function.
If we add another image view in the middle, then here's the vertical constraints we need to set (colored in Red, A, C, D, E and G) :
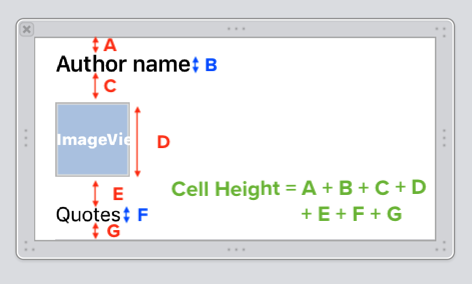
Image view without an image selected has no intrinsic content size, hence we need to set an explicit height constraint for it.
The key to achieve dynamic height table view cell is to let Auto Layout know how to calculate the height of the cell.
This article is an excerpt from the book Making Sense of Auto Layout , if you feel like you have no idea what you are doing when adding / removing / editing constraints, give the sample chapter a try!
Download the sample dynamic cell height project
Not sure if you are doing it right on the self sizing cell height?
Download the sample project and compare it yourself!
https://github.com/fluffyes/dynamicHeightTable